A quick spell I made in a few hours. Uses only like 66 lines plus lots of object editor stuff. I thought it was cool 
Plus, it's not super overpowered. (It seems like it is, but imagine holding the knights in the DoT field for as long as you can with 10000000 life
It may be a bit OP against summoned units because of the purge, but I haven't tested that so any input on that is welcome.
And, I'm learning cJASS, so here's a cJASS version:
And yes, cJASS version has a lot more comments.
Screenie:
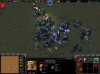
NOTE: Test is just a plain JASS version.
Comments?
Suggestions?
Plus, it's not super overpowered. (It seems like it is, but imagine holding the knights in the DoT field for as long as you can with 10000000 life
It may be a bit OP against summoned units because of the purge, but I haven't tested that so any input on that is welcome.
JASS:
/* Spell Information
===================================================================
Name: Lightning Totem
Version: 1.0
Author: Sevion
Requirements: NewGen v1.5d+
Installation:
Copy Hero and Abilities over to your map adn the code.
Setup:
Edit SPELL_ID, DUMMY_ID, MODEL_ID and DURATION.
Description:
------------------------------------------------------------------
Creates a totem at the target area that spews lightning at enemies
in the immediate area dealing damage, deals damage over time to
enemies, and casts purge on all units in range. Issueing an order
will cause the spell to disrupt and only the totem will be summoned,
no damage over time or purge will ensue. The area damage disperses
before the totem does and lasts only 3 seconds plus 1 for each level.
*/
scope LightningTotem initializer Ini
globals
constant integer SPELL_ID = 039;AHfs039;
constant integer DUMMY_ID = 039;ltdu039;
constant string MODEL_ID = "units\\orc\\StasisTotem\\StasisTotem.mdl"
endglobals
private constant function DURATION takes integer lvl returns real
return 10. + 5 * lvl
endfunction
private function PolledWaitCustom takes real duration returns nothing
local timer t=CreateTimer()
local real timeRemaining
if(duration>0) then
call TimerStart(t,duration,false,null)
loop
set timeRemaining=TimerGetRemaining(t)
exitwhen timeRemaining<=0
if(timeRemaining>bj_POLLED_WAIT_SKIP_THRESHOLD) then
call TriggerSleepAction(0.1*timeRemaining)
else
call TriggerSleepAction(bj_POLLED_WAIT_INTERVAL)
endif
endloop
endif
call DestroyTimer(t)
set t=null
endfunction
private function onSpell takes nothing returns nothing
local effect e
local unit u
if GetSpellAbilityId()==SPELL_ID then
set e=AddSpecialEffect(MODEL_ID,GetSpellTargetX(),GetSpellTargetY())
set u=CreateUnit(GetOwningPlayer(GetTriggerUnit()),DUMMY_ID,GetSpellTargetX(),GetSpellTargetY(),0)
call PolledWaitCustom(DURATION(GetUnitAbilityLevel(GetTriggerUnit(),SPELL_ID)))
call RemoveUnit(u)
call DestroyEffect(e)
set e=null
set u=null
endif
endfunction
private function Ini takes nothing returns nothing
local trigger trig=CreateTrigger()
local integer i=0x00
call TriggerAddAction(trig,function onSpell)
loop
call TriggerRegisterPlayerUnitEvent(trig,Player(i),EVENT_PLAYER_UNIT_SPELL_EFFECT,null)
set i=i+1
exitwhen i==0x10
endloop
set trig=null
call Preload(MODEL_ID)
call PreloadEnd(.5)
endfunction
endscope
scope Testing initializer Ini
private function Ini takes nothing returns nothing
call Cheat("iseedeadpeople")
call Cheat("thereisnospoon")
call CreateUnit(Player(0x00),039;Hblm039;,0,0,0)
call PanCameraTo(0,0)
endfunction
endscope
And, I'm learning cJASS, so here's a cJASS version:
And yes, cJASS version has a lot more comments.
JASS:
/* Spell Information
===================================================================
Name: Lightning Totem
Version: 1.0
Author: Sevion
Requirements: NewGen v1.5d+, cJASS
Installation:
Copy Hero and Abilities over to your map adn the code.
Setup:
Edit SPELL_ID, DUMMY_ID, MODEL_ID and DURATION.
Description:
------------------------------------------------------------------
Creates a totem at the target area that spews lightning at enemies
in the immediate area dealing damage, deals damage over time to
enemies, and casts purge on all units in range. Issueing an order
will cause the spell to disrupt and only the totem will be summoned,
no damage over time or purge will ensue. The area damage disperses
before the totem does and lasts only 3 seconds plus 1 for each level.
*/
scope LightningTotem initializer Ini {
//==============================
// defines
define {
private void = nothing
private int = integer
private bool = boolean
private float = real
private DisplayText ( text ) = DisplayTextToPlayer(GetLocalPlayer(), 0, 0, text)
private SPELL_ID = 039;AHfs039;
private DUMMY_ID = 039;ltdu039;
private MODEL_ID = "units\\orc\\StasisTotem\\StasisTotem.mdl"
private DURATION ( hero ) = 10. + 5 * GetUnitAbilityLevel( hero, SPELL_ID )
}
//=============================
// spell
private void PolledWaitCustom ( real duration ) {
timer t = CreateTimer()
float timeRemaining
if (duration > 0) {
TimerStart(t, duration, false, null)
loop {
timeRemaining = TimerGetRemaining(t)
exitwhen timeRemaining <= 0
if (timeRemaining > bj_POLLED_WAIT_SKIP_THRESHOLD) {
TriggerSleepAction(0.1 * timeRemaining)
else
TriggerSleepAction(bj_POLLED_WAIT_INTERVAL)
}
}
}
DestroyTimer(t)
set t = null
}
private void onSpell () {
if GetSpellAbilityId() == SPELL_ID {
effect e = AddSpecialEffect(MODEL_ID, GetSpellTargetX(), GetSpellTargetY())
unit u = CreateUnit(GetOwningPlayer(GetTriggerUnit()), DUMMY_ID, GetSpellTargetX(), GetSpellTargetY(), 0)
PolledWaitCustom(DURATION(GetTriggerUnit()))
RemoveUnit(u)
DestroyEffect(e)
e = null
u = null
}
}
// end lightning totem spell
//============================
//============================
// init
private void Ini () {
trigger trig = CreateTrigger() ; TriggerAddAction(trig, function onSpell)
int i = 0x00
loop {
TriggerRegisterPlayerUnitEvent(trig, Player(i), EVENT_PLAYER_UNIT_SPELL_EFFECT, null)
exitwhen ++i == 0x10
}
set trig = null
Preload(MODEL_ID)
PreloadEnd(.5)
}
// end init
//============================================
}
scope Testing initializer Ini {
private nothing Ini () {
Cheat("iseedeadpeople")
Cheat("thereisnospoon")
CreateUnit(Player(0x00), 039;Hblm039;, 0, 0, 0)
PanCameraTo(0, 0)
}
}
Screenie:
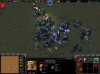
NOTE: Test is just a plain JASS version.
Comments?
Suggestions?