Wratox1
Member
- Reaction score
- 22
Hello, i've just started to enjoy programming java, and im currently makeing a small game.
but i've run into a problem where a jpanel does not display properly(unless i do mainFrame.pack(), but then the window is resized to a very small window) after i added components to it, set it visible, hide it, removed the compononents, and then add components and set it visible again.
at the moment i do remove all the components from the jpanel, but its only one component that needs to be removed, and then added again. I need to add, remove and then add this component again because its a jbutton which i reuse in 2 jpanels that are not displayed at the same time.
here is my code where i remove the jbutton called exitButton from the jpanel called mainMenuPane and then add it and the other components to the jpanel called menuPane(it's menuPane that is not displaying properly)
and here i remove the jbutton from menuPane and add it to mainMenuPane
here is a screenshot of how the jpanel looks when i've added the components, removed them and added them again:
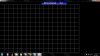
and here is how it should look:
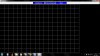
anyone know why the jpanel displays like it does?
tell me if you want more code, but its about 500 lines of code(including empty lines), just so you know.
//wratox
but i've run into a problem where a jpanel does not display properly(unless i do mainFrame.pack(), but then the window is resized to a very small window) after i added components to it, set it visible, hide it, removed the compononents, and then add components and set it visible again.
at the moment i do remove all the components from the jpanel, but its only one component that needs to be removed, and then added again. I need to add, remove and then add this component again because its a jbutton which i reuse in 2 jpanels that are not displayed at the same time.
here is my code where i remove the jbutton called exitButton from the jpanel called mainMenuPane and then add it and the other components to the jpanel called menuPane(it's menuPane that is not displaying properly)
Code:
private void createGameGraphics()
{
mainMenuPane.removeAll();
exitButton.setPreferredSize(new Dimension(150, 25));
exitButton.setMaximumSize(exitButton.getPreferredSize()) ;
menuPane.add(optionsButton);
menuPane.add(exitToMenuButton);
menuPane.add(exitButton);
mainFrame.add(menuPane);
graphicsPane.add(graphicsObject);
mainFrame.add(graphicsPane);
this.revalidate();
t.start();
}
and here i remove the jbutton from menuPane and add it to mainMenuPane
Code:
if ("Exit to Menu".equals(e.getActionCommand()))
{
t.stop();
mainMenuShow();
playGame = false;
menuPane.removeAll();
exitButton.setPreferredSize(new Dimension(200, 50));
exitButton.setMaximumSize(exitButton.getPreferredSize()) ;
mainFrame.remove(menuPane);
mainFrame.remove(graphicsPane);
}
Code:
public void mainMenuShow()
{
mainMenuPane.setOpaque(true); //content panes must be opaque
mainFrame.setContentPane(mainMenuPane);
mainMenuPane.setBackground ( Color.black);
mainMenuPane.setLayout(new BoxLayout( mainMenuPane, BoxLayout.Y_AXIS ) );
//mainMenuPane.add(Box.createVerticalGlue()); = creates a glue, an invisible box
mainMenuPane.add(Box.createVerticalGlue());
mainMenuPane.add(Box.createVerticalGlue());
mainMenuPane.add(playButton);
mainMenuPane.add(exitButton);
mainMenuPane.add(Box.createVerticalGlue());
mainMenuPane.add(Box.createVerticalGlue());
mainMenuPane.add(Box.createVerticalGlue());
mainMenuPane.add(Box.createVerticalGlue());
this.revalidate();
}
here is a screenshot of how the jpanel looks when i've added the components, removed them and added them again:
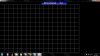
and here is how it should look:
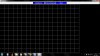
anyone know why the jpanel displays like it does?
tell me if you want more code, but its about 500 lines of code(including empty lines), just so you know.
//wratox