Andrewgosu
The Silent Pandaren Helper
- Reaction score
- 716
Multiboard I
a multiboard, which stores team score and flag captures, loses. Mainly for capture the flag type of maps.
a multiboard, which stores team score and flag captures, loses. Mainly for capture the flag type of maps.
Frozen Throne required
Step 1 - Multiboard initialization
cc_players - string variable array
cc_endtag - string variable
string_owns_flag - string variable
player_playing - player group variable
This initialization is basically the same as the previous one, but one extra variable is set and the cc_players string variable arrays have changed.
Code:
Multiboard Initialization
Events
Map initialization
Conditions
Actions
Set players_playing = (All players matching (((Matching player) slot status) Equal to Is playing))
-------- Gold colour --------
Set cc_players[0] = |cffffcc00
-------- Player colours. --------
Set cc_players[1] = |c00ff0303
Set cc_players[2] = |c000042ff
Set cc_players[3] = |c001ce6b9
Set cc_players[4] = |c00540081
Set cc_players[5] = |c00fffc01
Set cc_players[6] = |c00feba0e
Set cc_players[7] = |c0020c000
Set cc_players[8] = |c00e55bb0
Set cc_endtag = |r
-------- String owns. --------
Set string_owns_flag[1] = yes
Set string_owns_flag[2] = no
Step 2 – Multiboard itself
multiboard_flag - multiboard variable
player_row - integer variable, initial value 4
player_colour - integer variable
player_flag_got - integer variable
player_flag_lost - integer variable
Firstly, create a multiboard with 3 columns and 4 + number of players in players_playing. Then set it into a variable. (If you have more than 1 multiboard on your map, then its wiser to set them all into variables. We'll practise this.)
Code:
Multiboard Flag
Events
Time - Elapsed game time is 0.00 seconds
Conditions
Actions
Multiboard - Create a multiboard with 3 columns and (4 + (Number of players in players_playing)) rows, titled Information
Set multiboard_flag = (Last created multiboard)
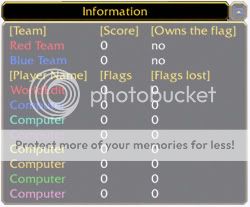
Then, set the text for row 1, column 1-3. For column 1, set it to Team, for column 2, set it to Score and for column 3, set it to Owns the flag.
Code:
-------- row 1, colum 1, 2, 3. --------
Multiboard - Set the text for multiboard_flag item in column 1, row 1 to (cc_players[0] + ([Team] + cc_endtag))
Multiboard - Set the text for multiboard_flag item in column 2, row 1 to (cc_players[0] + ([Score] + cc_endtag))
Multiboard - Set the text for multiboard_flag item in column 3, row 1 to (cc_players[0] + ([Owns the flag] + cc_endtag))
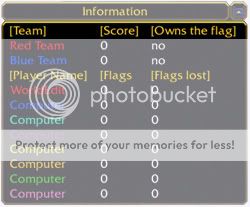
After that set the text and values for row 2, column 1-3, for the red team,
Code:
-------- row 2, colum 1, 2, 3. --------
-------- red team --------
Multiboard - Set the text for multiboard_flag item in column 1, row 2 to (cc_players[1] + (Red Team + cc_endtag))
Multiboard - Set the text for multiboard_flag item in column 2, row 2 to (String(team_score[1]))
Multiboard - Set the text for multiboard_flag item in column 3, row 2 to string_owns_flag[2]
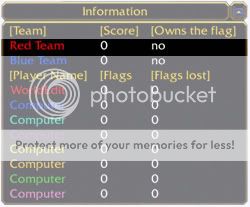
,and for row 3, column 1-3, for the blue team.
Code:
-------- row 3, colum 1, 2, 3. --------
-------- blue team --------
Multiboard - Set the text for multiboard_flag item in column 1, row 3 to (cc_players[2] + (Blue Team + cc_endtag))
Multiboard - Set the text for multiboard_flag item in column 2, row 3 to (String(team_score[2]))
Multiboard - Set the text for multiboard_flag item in column 3, row 3 to string_owns_flag[2]
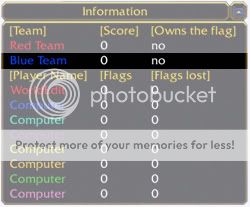
Now, one final row has to be set, before we begin to add players and set the multiboard width and style. Set the text for row 4, column 1 to Player name, for column 2 to flags obtained and for column 3, flags lost.
Code:
-------- row 4, colum 1, 2, 3. --------
Multiboard - Set the text for multiboard_flag item in column 1, row 4 to (cc_players[0] + ([Player Name] + cc_endtag))
Multiboard - Set the text for multiboard_flag item in column 2, row 4 to (cc_players[0] + ([Flags obtained] + cc_endtag))
Multiboard - Set the text for multiboard_flag item in column 3, row 4 to (cc_players[0] + ([Flags lost] + cc_endtag))
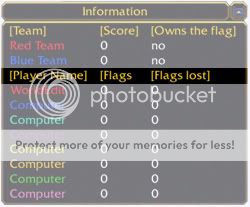
Now, the loop part. Firstly, change the multiboard style to hide all icons.
Code:
-------- loop part. --------
For each (Integer A) from 1 to (4 + (Number of players in players_playing)), do (Actions)
Loop - Actions
-------- Multiboard style. All icons have been hidden. --------
Multiboard - Set the display style for multiboard_flag item in column 1, row (Integer A) to Show text and Hide icons
Multiboard - Set the display style for multiboard_flag item in column 2, row (Integer A) to Show text and Hide icons
Multiboard - Set the display style for multiboard_flag item in column 3, row (Integer A) to Show text and Hide icons
Then, set the width.
Code:
-------- Multiboard width. --------
Multiboard - Set the width for multiboard_flag item in column 1, row (Integer A) to 9.00% of the total screen width
Multiboard - Set the width for multiboard_flag item in column 2, row (Integer A) to 5.00% of the total screen width
Multiboard - Set the width for multiboard_flag item in column 3, row (Integer A) to 9.00% of the total screen width
After that, add the players.
Code:
-------- Player adding part. --------
-------- Player name. --------
Set player_row = (player_row + 1)
Set player_colour = (player_colour + 1)
Multiboard - Set the text for multiboard_flag item in column 1, row player_row to (cc_players[player_colour] + ((Name of (Player(player_colour))) + cc_endtag))
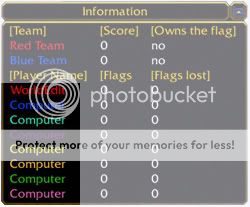
Then, just like adding the kills variable for the previous multiboard, we'll add the player_flag_got variable, representing the amount of flags captured, to the multiboard,
Code:
-------- Player flags. --------
Multiboard - Set the text for multiboard_flag item in column 2, row player_row to (String(player_flag_got[player_colour]))
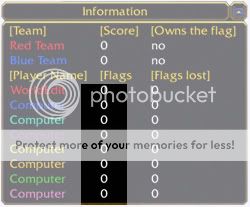
, and also the player_flag_lost variable, representing the amount of flags stolen from the player, to the multiboard.
Code:
-------- Player flags lost --------
Multiboard - Set the text for multiboard_flag item in column 3, row player_row to (String(player_flag_lost[player_colour]))
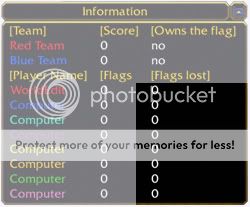
Lastly, show the multiboard. You should see something like this.
Code:
Multiboard Flag
Events
Time - Elapsed game time is 0.00 seconds
Conditions
Actions
Multiboard - Create a multiboard with 3 columns and (4 + (Number of players in players_playing)) rows, titled Information
Set multiboard_flag = (Last created multiboard)
-------- row 1, colum 1, 2, 3. --------
Multiboard - Set the text for multiboard_flag item in column 1, row 1 to (cc_players[0] + ([Team] + cc_endtag))
Multiboard - Set the text for multiboard_flag item in column 2, row 1 to (cc_players[0] + ([Score] + cc_endtag))
Multiboard - Set the text for multiboard_flag item in column 3, row 1 to (cc_players[0] + ([Owns the flag] + cc_endtag))
-------- row 2, colum 1, 2, 3. --------
-------- red team --------
Multiboard - Set the text for multiboard_flag item in column 1, row 2 to (cc_players[1] + (Red Team + cc_endtag))
Multiboard - Set the text for multiboard_flag item in column 2, row 2 to (String(team_score[1]))
Multiboard - Set the text for multiboard_flag item in column 3, row 2 to string_owns_flag[2]
-------- row 3, colum 1, 2, 3. --------
-------- blue team --------
Multiboard - Set the text for multiboard_flag item in column 1, row 3 to (cc_players[2] + (Blue Team + cc_endtag))
Multiboard - Set the text for multiboard_flag item in column 2, row 3 to (String(team_score[2]))
Multiboard - Set the text for multiboard_flag item in column 3, row 3 to string_owns_flag[2]
-------- row 4, colum 1, 2, 3. --------
Multiboard - Set the text for multiboard_flag item in column 1, row 4 to (cc_players[0] + ([Player Name] + cc_endtag))
Multiboard - Set the text for multiboard_flag item in column 2, row 4 to (cc_players[0] + ([Flags obtained] + cc_endtag))
Multiboard - Set the text for multiboard_flag item in column 3, row 4 to (cc_players[0] + ([Flags lost] + cc_endtag))
-------- loop part. --------
For each (Integer A) from 1 to (4 + (Number of players in players_playing)), do (Actions)
Loop - Actions
-------- Multiboard style. All icons have been hidden. --------
Multiboard - Set the display style for multiboard_flag item in column 1, row (Integer A) to Show text and Hide icons
Multiboard - Set the display style for multiboard_flag item in column 2, row (Integer A) to Show text and Hide icons
Multiboard - Set the display style for multiboard_flag item in column 3, row (Integer A) to Show text and Hide icons
-------- Multiboard width. --------
Multiboard - Set the width for multiboard_flag item in column 1, row (Integer A) to 9.00% of the total screen width
Multiboard - Set the width for multiboard_flag item in column 2, row (Integer A) to 5.00% of the total screen width
Multiboard - Set the width for multiboard_flag item in column 3, row (Integer A) to 9.00% of the total screen width
-------- Player adding part. --------
-------- Player name. --------
Set player_row = (player_row + 1)
Set player_colour = (player_colour + 1)
Multiboard - Set the text for multiboard_flag item in column 1, row player_row to (cc_players[player_colour] + ((Name of (Player(player_colour))) + cc_endtag))
-------- Player flags. --------
Multiboard - Set the text for multiboard_flag item in column 2, row player_row to (String(player_flag_got[player_colour]))
-------- Player flags lost --------
Multiboard - Set the text for multiboard_flag item in column 3, row player_row to (String(player_flag_lost[player_colour]))
Multiboard - Show multiboard_flag
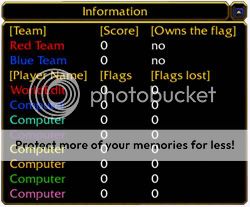
Step 3 - updating the values
For capture the flag, ther can be alot of events for updating the values. Make your own trigger with events and update the values just like with the first multiboard.
If team red captures the flag, change the leaderboard 'owns the flag' to 'yes' for team red and if the team, who captured the flag was blue, change it 'yes' fro blue team. If a team loses the flag, change it to 'no'.
If a player captures the flag, add +1 to his 'capture score' and +1 to the 'flag lost' score to the player, whom he got it from.
If a hero in team red enters to red base with the blue flag, set the red team score to +1. (The same for blue, if blue enters to its base with the red flag).
(Your events and conditions) For the 'captures the flag' and from whom the player captured it.
Code:
Player Flag Obtains
Events
Conditions
Actions
-------- Who captured the flag --------
Set player_flag_got[(Player number of (Owner of (Casting unit)))] = ((Player number of (Owner of (Casting unit))) + 1)
Multiboard - Set the text for multiboard_flag item in column 2, row (4 + (Player number of (Owner of (Casting unit)))) to (String(player_flag_got[(Player number of (Owner of (Casting unit)))]))
-------- From whom the flag was stolen --------
Set player_flag_lost[(Player number of (Owner of (Target unit of ability being cast)))] = (player_flag_lost[(Player number of (Owner of (Target unit of ability being cast)))] + 1)
Multiboard - Set the text for multiboard_flag item in column 3, row (4 + (Player number of (Owner of (Target unit of ability being cast)))) to (String(player_flag_lost[(Player number of (Owner of (Target unit of ability being cast)))]))
For overall team score. This one is for red, make another for blue. (your condtions and events)
Code:
Red score
Events
Conditions
Actions
Set team_score[1] = (team_score[1] + 1)
Multiboard - Set the text for multiboard_flag item in column 2, row 2 to (String(team_score[1]))