Random Spells
By kenny!
v3
By kenny!
v3
Elemental Helix
JASS: Yes, vJass.
JESP: Yes.
Leakless: Yes.
Lagless: Yes.
MUI: Yes.
Requires: NewGen and GTrigger.
Description:
Found in my next post (Post #4).
Screenshot:
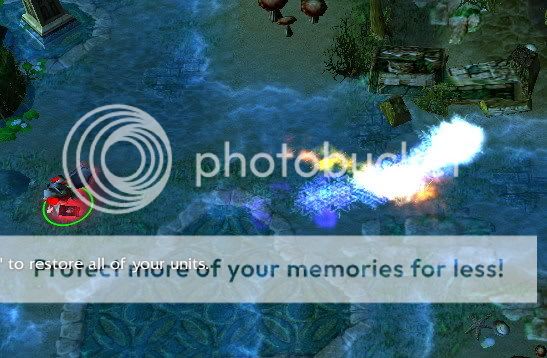
Script:
JASS:
//------------------------------------------------------------------------------------\\
// Elemental Helix [v3] \\
// by kenny! \\
// Constructed using vJASS \\
// Requires NewGen WE & GTrigger \\
//------------------------------------------------------------------------------------\\
scope ElementalHelix2
globals
// Configurables:
// Raw codes of units and spells:
private constant integer ABIL_ID = 039;A000039; // Raw code of Elemental Helix ability.
private constant integer ABIL2_ID = 039;A005039; // Raw code of Entangling Roots ability (freeze).
private constant integer CROW_FORM = 039;Amrf039; // Raw code of the standard Crow Form ability.
private constant integer FROST_ID = 039;n000039; // Raw code of Elemental Helix dummy unit (ICE).
private constant integer FLAME_ID = 039;n001039; // Raw code of Elemental Helix dummy unit (FIRE).
private constant integer DUMMY_ID = 039;u001039; // Raw code of the dummy unit that casts entangling roots (freeze).
// Movement, speed and size of orbs:
private constant real INTERVAL = 0.03 // INTERVAL used for the periodic timer, 0.03 - 0.04 is recommended.
private constant real COLLISION = 128.00 // How close units have to be to get hit by the projectiles.
private constant real MOVE_DIST = 22.50 // Distance moved per interval/period (INTERVAL), higher the number = faster movement
private constant real PARTIAL_ANGLE = 12.00 // Partial angle that is need to create 'Helix' effect, best kept at 12.00, or between 11.00-13.00
private constant real ORB_WIDTH = 50.00 // Used as distance for dummy units to move in and out, 50.00 is good, higher creates a wider gap, opposite for lower
private constant real FROST_SIZE = 0.60 // Size of the Ice orb
private constant real FLAME_SIZE = 1.10 // Size of the Fire orb
private constant real FLY_HEIGHT = 45.00 // Fly height of both of the orbs, good height is anything between 10.00-50.00
private constant real START_DIST = 35.00 // Distance away from the caster at which both orbs will be created
// Attack and damage types:
private constant attacktype A_TYPE = ATTACK_TYPE_CHAOS // Attack type for the Fire damage and the end explosion.
private constant damagetype D_TYPE = DAMAGE_TYPE_UNIVERSAL // Damage type for the Fire damage and the end explosion.
private constant weapontype W_TYPE = WEAPON_TYPE_WHOKNOWS // Weapon type for the Fire damage and the end explosion.
// Special effects and attachments:
private constant string FLAME_HIT = "Environment\\LargeBuildingFire\\LargeBuildingFire2.mdl" // Used for the fire damage effect on units.
private constant string FROST_HIT = "Abilities\\Spells\\Undead\\FrostArmor\\FrostArmorDamage.mdl" // Used for the ice effect on units.
private constant string END_FLAME = "Objects\\Spawnmodels\\Other\\NeutralBuildingExplosion\\NeutralBuildingExplosion.mdl" // Used for fire end explosion.
private constant string END_FROST = "Abilities\\Spells\\Undead\\FrostNova\\FrostNovaTarget.mdl" // Used for ice end explosion.
private constant string COMBUST_SFX = "Abilities\\Spells\\Other\\Doom\\DoomDeath.mdl" // Used for combustion effect when the fire orb hits.
private constant string ATTACH_POINT = "chest" // Used to attach fire and ice effects to enemy target units (FLAME_HIT and FROST_HIT).
// Others:
private constant real TIMED_LIFE = 1.00 // Timed life for caster units for entangle; Can be changed if necessary.
private constant boolean DESTROY_TREES = true // Whether or not to allow destroying trees in end explosion.
private constant boolean ALLOW_PRELOAD = true // Whether or not to allow preloading of effects.
private constant string ORDER_STRING = "entanglingroots" // Order string for the freeze ability (Abil2_id).
endglobals
private function Flame_damage takes integer lvl returns real
return 50.00 * lvl // Damage dealt to enemy units that come close to the fire dummy.
endfunction
private function End_damage takes integer lvl returns real
return 50.00 + (50.00 * lvl) // Damage dealt to enemy units at the end explosion.
endfunction
private function End_radius takes integer lvl returns real
return 150.00 + (50.00 * lvl) // Area of Effect for the end explosion.
endfunction
private function Combust_chance takes integer lvl returns integer
return 10 + (5 * lvl) // Percent chance for the combustion when the fireball its an enemy unit.
endfunction
private function Combust_radius takes integer lvl returns real
return 50.00 * lvl // Area of effect of the combustion when the fireball hits.
endfunction
private function Combust_damage takes integer lvl returns real
return 25.00 * lvl // Damage dealt by the combustion when it hits.
endfunction
//=======================================================================\\
// DO NOT TOUCH PAST THIS POINT UNLESS YOU KNOW WHAT YOUR ARE DOING! \\
//=======================================================================\\
private struct Data
unit cast = null
unit dummy1 = null
unit dummy2 = null
unit dummy3 = null
real targx = 0.00
real targy = 0.00
real angle = 0.00
real newangle1 = 0.00
real newangle2 = 0.00
real sin = 0.00
real cos = 0.00
real x = 0.00
real y = 0.00
real start1x = 0.00
real start1y = 0.00
real start2x = 0.00
real start2y = 0.00
real maxdist = 0.00
integer level = 0
real dist = START_DIST
group frost = CreateGroup()
group flame = CreateGroup()
static Data array D
static integer DT = 0
static rect Rect1 = null
static timer Timer = null
static group Group1 = null
static group Group2 = null
static unit Tree_dummy = null
static boolexpr Tree_filt = null
static boolexpr Enemy_filt = null
static real Game_maxX = 0.00
static real Game_minX = 0.00
static real Game_maxY = 0.00
static real Game_minY = 0.00
static method safex takes real x returns real
if x < .Game_minX then
return .Game_minX
elseif x > .Game_maxX then
return .Game_maxX
endif
return x
endmethod
static method safey takes real y returns real
if y < .Game_minY then
return .Game_minY
elseif y > .Game_maxY then
return .Game_maxY
endif
return y
endmethod
static method destroyenumtrees takes nothing returns nothing
call KillDestructable(GetEnumDestructable())
endmethod
static method treefilt takes nothing returns boolean
local destructable dest = GetFilterDestructable()
local boolean result = false
if GetDestructableLife(dest) > 0.405 then
call ShowUnit(.Tree_dummy,true)
call SetUnitX(.Tree_dummy,GetWidgetX(dest))
call SetUnitY(.Tree_dummy,GetWidgetY(dest))
set result = IssueTargetOrder(.Tree_dummy,"harvest",dest)
call IssueImmediateOrder(.Tree_dummy,"stop")
call ShowUnit(.Tree_dummy,false)
set dest = null
return result
endif
set dest = null
return result
endmethod
static method enemyfilt takes nothing returns boolean
return GetWidgetLife(GetFilterUnit()) > 0.405 and IsUnitType(GetFilterUnit(),UNIT_TYPE_STRUCTURE) == false and IsUnitType(GetFilterUnit(),UNIT_TYPE_FLYING) == false
endmethod
private method onDestroy takes nothing returns nothing
local unit u = null
call GroupEnumUnitsInRange(.Group1,.targx,.targy,End_radius(.level),.Enemy_filt)
loop
set u = FirstOfGroup(.Group1)
exitwhen u == null
call GroupRemoveUnit(.Group1,u)
if IsUnitEnemy(u,GetOwningPlayer(.cast)) then
set .dummy3 = CreateUnit(GetOwningPlayer(.cast),DUMMY_ID,.targx,.targy,0.00)
call UnitDamageTarget(.cast,u,End_damage(.level),false,false,A_TYPE,D_TYPE,W_TYPE)
call UnitAddAbility(.dummy3,ABIL2_ID)
call SetUnitAbilityLevel(.dummy3,ABIL2_ID,.level)
call UnitApplyTimedLife(.dummy3,039;BTLF039;,TIMED_LIFE)
call IssueTargetOrder(.dummy3,ORDER_STRING,u)
set .dummy3 = null
endif
endloop
if DESTROY_TREES then
call SetRect(.Rect1,.targx - End_radius(.level),.targy - End_radius(.level),.targx + End_radius(.level),.targy + End_radius(.level))
call EnumDestructablesInRect(.Rect1,.Tree_filt,function Data.destroyenumtrees)
endif
call DestroyGroup(.frost)
call DestroyGroup(.flame)
set .frost = null
set .flame = null
call DestroyEffect(AddSpecialEffect(END_FROST,GetUnitX(.dummy1),GetUnitY(.dummy1)))
call DestroyEffect(AddSpecialEffect(END_FLAME,GetUnitX(.dummy2),GetUnitY(.dummy2)))
call KillUnit(.dummy1)
call KillUnit(.dummy2)
set .cast = null
set .dummy1 = null
set .dummy2 = null
set .dummy3 = null
set u = null
endmethod
static method update takes nothing returns nothing
local Data d = 0
local integer i = 1
local real x = 0.00
local real y = 0.00
local unit u = null
local unit n = null
loop
exitwhen i > .DT
set d = .D<i>
if d.dist >= d.maxdist then
call d.destroy()
set .D<i> = .D[.DT]
set .DT = .DT - 1
set i = i - 1
else
set d.x = d.x + MOVE_DIST * d.cos
set d.y = d.y + MOVE_DIST * d.sin
set d.newangle1 = d.newangle1 + PARTIAL_ANGLE
set d.newangle2 = d.newangle2 + PARTIAL_ANGLE
set x = .safex(d.x + ORB_WIDTH * Cos(d.newangle1 * bj_DEGTORAD))
set y = .safey(d.y + ORB_WIDTH * Sin(d.newangle1 * bj_DEGTORAD))
call SetUnitPosition(d.dummy1,x,y)
call GroupEnumUnitsInRange(.Group1,x,y,COLLISION,.Enemy_filt)
loop
set u = FirstOfGroup(.Group1)
exitwhen u == null
call GroupRemoveUnit(.Group1,u)
if not IsUnitInGroup(u,d.frost) and IsUnitEnemy(u,GetOwningPlayer(d.cast)) then
call GroupAddUnit(d.frost,u)
call DestroyEffect(AddSpecialEffectTarget(FROST_HIT,u,ATTACH_POINT))
set d.dummy3 = CreateUnit(GetOwningPlayer(d.cast),DUMMY_ID,x,y,0.00)
call UnitAddAbility(d.dummy3,ABIL2_ID)
call SetUnitAbilityLevel(d.dummy3,ABIL2_ID,d.level)
call UnitApplyTimedLife(d.dummy3,039;BTLF039;,TIMED_LIFE)
call IssueTargetOrder(d.dummy3,ORDER_STRING,u)
set d.dummy3 = null
endif
endloop
set x = .safex(d.x + ORB_WIDTH * Cos(d.newangle2 * bj_DEGTORAD))
set y = .safey(d.y + ORB_WIDTH * Sin(d.newangle2 * bj_DEGTORAD))
call SetUnitPosition(d.dummy2,x,y)
call GroupEnumUnitsInRange(.Group1,x,y,COLLISION,.Enemy_filt)
loop
set u = FirstOfGroup(.Group1)
exitwhen u == null
call GroupRemoveUnit(.Group1,u)
if not IsUnitInGroup(u,d.flame) and IsUnitEnemy(u,GetOwningPlayer(d.cast)) then
call GroupAddUnit(d.flame,u)
call UnitDamageTarget(d.cast,u,Flame_damage(d.level),false,false,A_TYPE,D_TYPE,W_TYPE)
call DestroyEffect(AddSpecialEffectTarget(FLAME_HIT,u,ATTACH_POINT))
if GetRandomInt(0,100) <= Combust_chance(d.level) then
call DestroyEffect(AddSpecialEffect(COMBUST_SFX,GetUnitX(u),GetUnitY(u)))
call GroupEnumUnitsInRange(.Group2,GetUnitX(u),GetUnitY(u),Combust_radius(d.level),.Enemy_filt)
loop
set n = FirstOfGroup(.Group2)
exitwhen n == null
call GroupRemoveUnit(.Group2,n)
call UnitDamageTarget(d.cast,n,Combust_damage(d.level),false,false,A_TYPE,D_TYPE,W_TYPE)
endloop
endif
endif
endloop
set d.dist = d.dist + MOVE_DIST
endif
set i = i + 1
endloop
if .DT <= 0 then
call PauseTimer(.Timer)
set .DT = 0
endif
set u = null
set n = null
endmethod
static method actions takes nothing returns boolean
local Data d = Data.create()
local location l = GetSpellTargetLoc()
local real angle = 0.00
local real castx = 0.00
local real casty = 0.00
set d.cast = GetTriggerUnit()
set castx = GetUnitX(d.cast)
set casty = GetUnitY(d.cast)
set d.targx = .safex(GetLocationX(l))
set d.targy = .safey(GetLocationY(l))
set angle = Atan2((d.targy - casty),(d.targx - castx)) * bj_RADTODEG
set d.cos = Cos(angle * bj_DEGTORAD)
set d.sin = Sin(angle * bj_DEGTORAD)
set d.x = GetUnitX(d.cast) + START_DIST * d.cos
set d.y = GetUnitY(d.cast) + START_DIST * d.sin
set d.start1x = d.x + START_DIST * Cos((angle + 90.00) * bj_DEGTORAD)
set d.start1y = d.y + START_DIST * Sin((angle + 90.00) * bj_DEGTORAD)
set d.start2x = d.x + START_DIST * Cos((angle - 90.00) * bj_DEGTORAD)
set d.start2y = d.y + START_DIST * Sin((angle - 90.00) * bj_DEGTORAD)
set d.newangle1 = angle + 90.00
set d.newangle2 = angle - 90.00
set d.dummy1 = CreateUnit(GetOwningPlayer(d.cast),FROST_ID,d.start1x,d.start1y,0.00)
set d.dummy2 = CreateUnit(GetOwningPlayer(d.cast),FLAME_ID,d.start2x,d.start2y,0.00)
set d.maxdist = SquareRoot((castx - d.targx) * (castx - d.targx) + (casty - d.targy) * (casty - d.targy))
set d.level = GetUnitAbilityLevel(d.cast,ABIL_ID)
call SetUnitScale(d.dummy1,FROST_SIZE,FROST_SIZE,FROST_SIZE)
call SetUnitScale(d.dummy2,FLAME_SIZE,FLAME_SIZE,FLAME_SIZE)
call UnitAddAbility(d.dummy1,CROW_FORM)
call UnitRemoveAbility(d.dummy1,CROW_FORM)
call UnitAddAbility(d.dummy2,CROW_FORM)
call UnitRemoveAbility(d.dummy2,CROW_FORM)
call SetUnitFlyHeight(d.dummy1,FLY_HEIGHT,0.00)
call SetUnitFlyHeight(d.dummy2,FLY_HEIGHT,0.00)
set .DT = .DT + 1
set .D[.DT] = d
if .DT == 1 then
call TimerStart(.Timer,INTERVAL,true,function Data.update)
endif
call RemoveLocation(l)
set l = null
return false
endmethod
static method onInit takes nothing returns nothing
set .Timer = CreateTimer()
set .Group1 = CreateGroup()
set .Group2 = CreateGroup()
set .Rect1 = Rect(0.00,0.00,1.00,1.00)
set .Tree_filt = Filter(function Data.treefilt)
set .Enemy_filt = Filter(function Data.enemyfilt)
set .Game_maxX = GetRectMaxX(bj_mapInitialPlayableArea) - 64.00
set .Game_maxY = GetRectMaxY(bj_mapInitialPlayableArea) - 64.00
set .Game_minX = GetRectMinX(bj_mapInitialPlayableArea) + 64.00
set .Game_minY = GetRectMinY(bj_mapInitialPlayableArea) + 64.00
// Register event.
call GT_AddStartsEffectAction(function Data.actions,ABIL_ID)
set .Tree_dummy = CreateUnit(Player(PLAYER_NEUTRAL_PASSIVE),DUMMY_ID,0.00,0.00,0.00)
call SetUnitPathing(.Tree_dummy,false)
call ShowUnit(.Tree_dummy,false)
if ALLOW_PRELOAD then
call DestroyEffect(AddSpecialEffect(FLAME_HIT,0.00,0.00))
call DestroyEffect(AddSpecialEffect(FROST_HIT,0.00,0.00))
call DestroyEffect(AddSpecialEffect(END_FLAME,0.00,0.00))
call DestroyEffect(AddSpecialEffect(END_FROST,0.00,0.00))
call DestroyEffect(AddSpecialEffect(COMBUST_SFX,0.00,0.00))
endif
endmethod
endstruct
endscope
</i></i>
Magnetic Field
JASS: Yes, vJass.
JESP: Yes.
Leakless: Yes.
Lagless: Yes.
MUI: Yes.
Requires: NewGen and GTrigger.
Description:
Found in my next post (Post #4).
Screenshot:
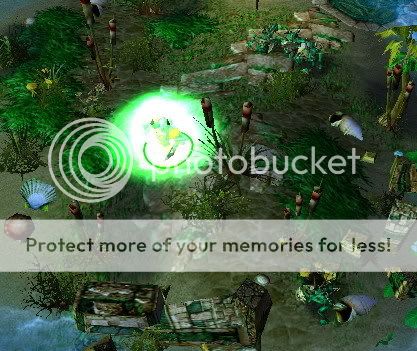
Script:
JASS:
//------------------------------------------------------------------------------------\\
// Magnetic Field [v3] \\
// by kenny! \\
// Constructed using vJASS \\
// Requires NewGen WE & GTrigger \\
//------------------------------------------------------------------------------------\\
scope MagneticField2
globals
// Configurables:
private constant integer ABIL_ID = 039;A001039; // Raw code of the Magnetic Field ability.
private constant integer DUMMY_ID = 039;u001039; // Raw code of the dummy unit used to destroy trees.
private constant real INTERVAL = 0.03125 // Used in the periodic timer to move units.
private constant real COLLISION = 150.00 // Area around the moving units in which trees will be destroyed.
private constant real DISTANCE = 75.00 // DISTANCE for checking pathability. Should be at least 25.00 distance less than COLLISION!!!
private constant string CASTED_SFX = "Abilities\\Spells\\Undead\\Darksummoning\\DarkSummonTarget.mdl" // Special effect that is attached to the caster.
private constant string CASTED_POINT = "origin" // where the special effect is attached to the caster.
private constant boolean DESTROY_TREES = true // Whether or not to allow destroying trees.
private constant boolean ALLOW_PRELOAD = true // Whether or not to allow preloading of effects.
endglobals
private function Duration takes integer lvl returns real
return 6.00 * lvl
endfunction
private function Move_dist takes integer lvl returns real
return 20.00 + (0.00 * lvl)
endfunction
private function Radius takes integer lvl returns real
return 225.00 + (50.00 * lvl)
endfunction
//=======================================================================\\
// DO NOT TOUCH PAST THIS POINT UNLESS YOU KNOW WHAT YOUR ARE DOING! \\
//=======================================================================\\
private struct Data
unit cast = null
effect sfx = null
real time = 0.00
integer lvl = 0
static Data array D
static item array Hidden
static integer Hidden_max = 0
static integer DT = 0
static rect Rect1 = null
static timer Timer = null
static group Group = null
static unit Tree_dummy = null
static item Item = null
static boolexpr Tree_filt = null
static boolexpr Unit_filt = null
static real Game_maxX = 0.00
static real Game_minX = 0.00
static real Game_maxY = 0.00
static real Game_minY = 0.00
static real Max_range = 10.00
static method hide takes nothing returns nothing
if IsItemVisible(GetEnumItem()) then
set .Hidden[.Hidden_max] = GetEnumItem()
call SetItemVisible(.Hidden[.Hidden_max],false)
set .Hidden_max = .Hidden_max + 1
endif
endmethod
static method pathability takes real x1, real y1 returns boolean
local real x2 = 0.00
local real y2 = 0.00
call SetRect(.Rect1,0.00,0.00,128.00,128.00)
call MoveRectTo(.Rect1,x1,y1)
call EnumItemsInRect(.Rect1,null,function Data.hide)
call SetItemPosition(.Item,x1,y1)
set x2 = GetItemX(.Item)
set y2 = GetItemY(.Item)
call SetItemVisible(.Item,false)
loop
exitwhen .Hidden_max <= 0
set .Hidden_max = .Hidden_max - 1
call SetItemVisible(.Hidden[.Hidden_max],true)
set .Hidden[.Hidden_max] = null
endloop
return (x1 - x2) * (x1 - x2) + (y1 - y2) * (y1 - y2) < .Max_range * .Max_range
endmethod
static method safex takes real x returns real
if x < .Game_minX then
return .Game_minX
elseif x > .Game_maxX then
return .Game_maxX
endif
return x
endmethod
static method safey takes real y returns real
if y < .Game_minY then
return .Game_minY
elseif y > .Game_maxY then
return .Game_maxY
endif
return y
endmethod
static method destroyenumtrees takes nothing returns nothing
call KillDestructable(GetEnumDestructable())
endmethod
static method treefilt takes nothing returns boolean
local destructable dest = GetFilterDestructable()
local boolean result = false
if GetDestructableLife(dest) > 0.405 then
call ShowUnit(.Tree_dummy,true)
call SetUnitX(.Tree_dummy,GetWidgetX(dest))
call SetUnitY(.Tree_dummy,GetWidgetY(dest))
set result = IssueTargetOrder(.Tree_dummy,"harvest",dest)
call IssueImmediateOrder(.Tree_dummy,"stop")
call ShowUnit(.Tree_dummy,false)
set dest = null
return result
endif
set dest = null
return result
endmethod
static method unitfilt takes nothing returns boolean
return GetWidgetLife(GetFilterUnit()) > 0.405 and IsUnitType(GetFilterUnit(),UNIT_TYPE_STRUCTURE) == false
endmethod
private method onDestroy takes nothing returns nothing
call DestroyEffect(.sfx)
set .sfx = null
set .cast = null
endmethod
static method update takes nothing returns nothing
local Data d = 0
local integer i = 1
local unit u = null
local real castx = 0.00
local real casty = 0.00
local real angle = 0.00
local real sin = 0.00
local real cos = 0.00
local real ux = 0.00
local real uy = 0.00
loop
exitwhen i > .DT
set d = .D<i>
set castx = GetUnitX(d.cast)
set casty = GetUnitY(d.cast)
if d.time >= Duration(d.lvl) or GetWidgetLife(d.cast) < 0.405 then
call d.destroy()
set .D<i> = .D[.DT]
set .DT = .DT - 1
set i = i - 1
else
call GroupEnumUnitsInRange(.Group,castx,casty,Radius(d.lvl),.Unit_filt)
call GroupRemoveUnit(.Group,d.cast)
loop
set u = FirstOfGroup(.Group)
exitwhen u == null
call GroupRemoveUnit(.Group,u)
set ux = GetUnitX(u)
set uy = GetUnitY(u)
set angle = Atan2((uy - casty),(ux - castx))
set sin = Sin(angle)
set cos = Cos(angle)
if .pathability(ux + DISTANCE * cos,uy + DISTANCE * sin) then
set ux = .safex(ux + Move_dist(d.lvl) * cos)
set uy = .safey(uy + Move_dist(d.lvl) * sin)
call SetUnitPosition(u,ux,uy)
if DESTROY_TREES then
call SetRect(.Rect1,ux - COLLISION,uy - COLLISION,ux + COLLISION,uy + COLLISION)
call EnumDestructablesInRect(.Rect1,.Tree_filt,function Data.destroyenumtrees)
endif
endif
endloop
set d.time = d.time + INTERVAL
endif
set i = i + 1
endloop
if .DT <= 0 then
call PauseTimer(.Timer)
set .DT = 0
endif
set u = null
endmethod
static method actions takes nothing returns boolean
local Data d = Data.create()
set d.cast = GetTriggerUnit()
set d.lvl = GetUnitAbilityLevel(d.cast,ABIL_ID)
set d.sfx = AddSpecialEffectTarget(CASTED_SFX,d.cast,CASTED_POINT)
set .DT = .DT + 1
set .D[.DT] = d
if .DT == 1 then
call TimerStart(.Timer,INTERVAL,true,function Data.update)
endif
return false
endmethod
static method onInit takes nothing returns nothing
set .Timer = CreateTimer()
set .Group = CreateGroup()
set .Rect1 = Rect(0.00,0.00,1.00,1.00)
set .Tree_filt = Filter(function Data.treefilt)
set .Unit_filt = Filter(function Data.unitfilt)
set .Game_maxX = GetRectMaxX(bj_mapInitialPlayableArea) - 64.00
set .Game_maxY = GetRectMaxY(bj_mapInitialPlayableArea) - 64.00
set .Game_minX = GetRectMinX(bj_mapInitialPlayableArea) + 64.00
set .Game_minY = GetRectMinY(bj_mapInitialPlayableArea) + 64.00
// Register event.
call GT_AddStartsEffectAction(function Data.actions,ABIL_ID)
set .Tree_dummy = CreateUnit(Player(PLAYER_NEUTRAL_PASSIVE),DUMMY_ID,0.00,0.00,0.00)
call SetUnitPathing(.Tree_dummy,false)
call ShowUnit(.Tree_dummy,false)
set .Item = CreateItem(039;ciri039;,0.00,0.00)
call SetItemVisible(.Item,false)
if ALLOW_PRELOAD then
call DestroyEffect(AddSpecialEffect(CASTED_SFX,0.00,0.00))
endif
endmethod
endstruct
endscope
</i></i>
Firewall
JASS: Yes, vJass.
JESP: Yes.
Leakless: Yes.
Lagless: Yes.
MUI: Yes.
Requires: NewGen and GTrigger.
Description:
Found in my next post (Post #4).
Screenshot:
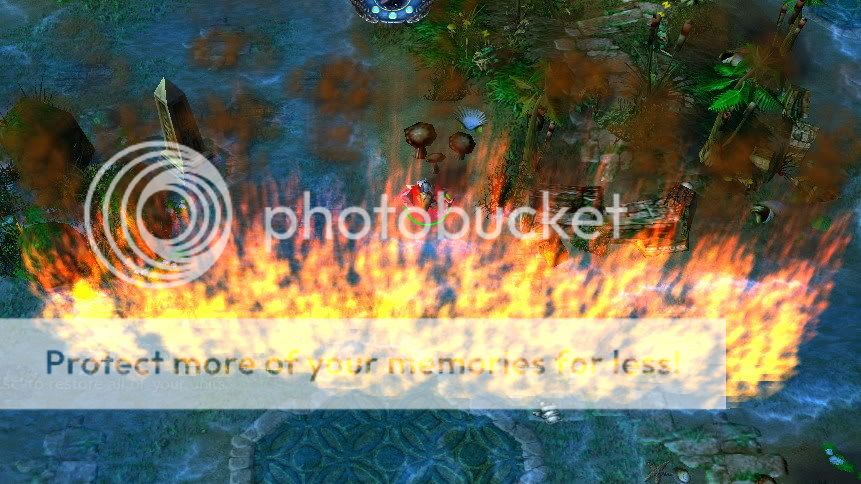
Script:
JASS:
//------------------------------------------------------------------------------------\\
// Firewall [v3] \\
// by kenny! \\
// Constructed using vJASS \\
// Requires NewGen WE & GTrigger \\
//------------------------------------------------------------------------------------\\
scope Firewall2
globals
private constant integer ABIL_ID = 039;A002039; // Raw code of the Firewall ability.
private constant integer ABIL2_ID = 039;A003039; // Raw code of the permanent immolation ability.
private constant integer UNIT_ID = 039;n002039; // Raw code of the dummy unit used for the fire wall.
private constant integer TREEDUMMY_ID = 039;u001039; // Raw code of the dummy unit used for destroying trees.
private constant real INTERVAL = 0.25 // INTERVAL for periodic timer, creating the wall over time. Try to keep it down around 0.02 - 0.30.
private constant real SPREAD_DIST = 125.00 // Distance between each part of the fire wall when they are created.
private constant real SCALE = 1.50 // Original scale size of the middle wall section.
private constant string BEGIN_SFX = "Abilities\\Spells\\Other\\Doom\\DoomDeath.mdl" // Effect played for the beginning explosion.
private constant string DAMAGE_SFX = "Abilities\\Weapons\\FireBallMissile\\FireBallMissile.mdl" // Effect played when a unit is hurt by that explosion.
private constant string DAMAGE_POINT = "chest" // Attachment point for above effect.
private constant attacktype A_TYPE = ATTACK_TYPE_CHAOS // Attack type of damage dealt by explosion.
private constant damagetype D_TYPE = DAMAGE_TYPE_UNIVERSAL // Damage type of damage dealt by explosion.
private constant weapontype W_TYPE = WEAPON_TYPE_WHOKNOWS // Weapon type of damage dealt by explosion.
private constant boolean DESTROY_TREES = true // Whether or not to destroy trees.
private constant boolean ALLOW_PRELOAD = true // Whether or not to allow preloading of effects.
endglobals
private function Max_length takes integer lvl returns integer
return 1 + (1 * lvl) // Legnth of the wall. This will be the number of wall sections created on each side of the middle section.
endfunction // For example: Level 3 = 4 sections either side, therefore 9 parts are created all together, at "SPREAD_DIST" distance apart from each other.
private function Duration takes integer lvl returns real
return 6.00 + (2.00 + lvl) // How long the wall will last.
endfunction
private function Radius takes integer lvl returns real
return 100.00 + (20.00 * lvl) // Radius of the explosion at the start.
endfunction
private function Damage takes integer lvl returns real
return 40.00 + (40.00 * lvl) // Damage dealt by beginning explosion.
endfunction
private function Scale_reduction takes integer spread returns real
return 0.10 * spread // SCALE reduction of the wall sections. Gives a nice effect (Large in middle - smaller on sides).
endfunction
//=======================================================================\\
// DO NOT TOUCH PAST THIS POINT UNLESS YOU KNOW WHAT YOUR ARE DOING! \\
//=======================================================================\\
private struct Data
unit cast = null
real castx = 0.00
real casty = 0.00
real locx = 0.00
real locy = 0.00
real sin1 = 0.00
real sin2 = 0.00
real cos1 = 0.00
real cos2 = 0.00
integer level = 0
integer spread = 0
static Data array D
static integer DT = 0
static rect Rect1 = null
static timer Timer = null
static group Group = null
static unit Tree_dummy = null
static boolexpr Tree_filt = null
static boolexpr Enemy_filt = null
static real Game_maxX = 0.00
static real Game_minX = 0.00
static real Game_maxY = 0.00
static real Game_minY = 0.00
static method safex takes real x returns real
if x < .Game_minX then
return .Game_minX
elseif x > .Game_maxX then
return .Game_maxX
endif
return x
endmethod
static method safey takes real y returns real
if y < .Game_minY then
return .Game_minY
elseif y > .Game_maxY then
return .Game_maxY
endif
return y
endmethod
static method destroyenumtrees takes nothing returns nothing
call KillDestructable(GetEnumDestructable())
endmethod
static method treefilt takes nothing returns boolean
local destructable dest = GetFilterDestructable()
local boolean result = false
if GetDestructableLife(dest) > 0.405 then
call ShowUnit(.Tree_dummy,true)
call SetUnitX(.Tree_dummy,GetWidgetX(dest))
call SetUnitY(.Tree_dummy,GetWidgetY(dest))
set result = IssueTargetOrder(.Tree_dummy,"harvest",dest)
call IssueImmediateOrder(.Tree_dummy,"stop")
call ShowUnit(.Tree_dummy,false)
set dest = null
return result
endif
set dest = null
return result
endmethod
static method enemyfilt takes nothing returns boolean
return GetWidgetLife(GetFilterUnit()) > 0.405 and IsUnitType(GetFilterUnit(),UNIT_TYPE_STRUCTURE) == false and IsUnitType(GetFilterUnit(),UNIT_TYPE_FLYING) == false
endmethod
private method onDestroy takes nothing returns nothing
set .cast = null
endmethod
static method update takes nothing returns nothing
local Data d = 0
local integer i = 1
local real radius = 0.00
local real newx1 = 0.00
local real newy1 = 0.00
local real newx2 = 0.00
local real newy2 = 0.00
local unit flame = null
local unit first = null
loop
exitwhen i > .DT
set d = .D<i>
set radius = Radius(d.level)
set d.spread = d.spread + 1
if d.spread > Max_length(d.level) then
call d.destroy()
set .D<i> = .D[.DT]
set .DT = .DT - 1
set i = i - 1
else
set newx1 = .safex(d.locx + (d.spread * SPREAD_DIST) * d.cos1)
set newy1 = .safey(d.locy + (d.spread * SPREAD_DIST) * d.sin1)
set newx2 = .safex(d.locx + (d.spread * SPREAD_DIST) * d.cos2)
set newy2 = .safey(d.locy + (d.spread * SPREAD_DIST) * d.sin2)
set flame = CreateUnit(GetOwningPlayer(d.cast),UNIT_ID,newx1,newy1,0.00)
call SetUnitScale(flame,(SCALE - Scale_reduction(d.spread)),(SCALE - Scale_reduction(d.spread)),0.00)
call DestroyEffect(AddSpecialEffect(BEGIN_SFX,newx1,newy1))
call UnitApplyTimedLife(flame,039;BTLF039;,Duration(d.level))
call SetUnitAbilityLevel(flame,ABIL2_ID,d.level)
call PauseUnit(flame,true)
call PauseUnit(flame,false)
call GroupEnumUnitsInRange(.Group,newx1,newy1,radius,.Enemy_filt)
loop
set first = FirstOfGroup(.Group)
exitwhen first == null
call GroupRemoveUnit(.Group,first)
if IsUnitEnemy(first,GetOwningPlayer(d.cast)) then
call UnitDamageTarget(d.cast,first,Damage(d.level),false,false,A_TYPE,D_TYPE,W_TYPE)
call DestroyEffect(AddSpecialEffectTarget(DAMAGE_SFX,first,DAMAGE_POINT))
endif
endloop
if DESTROY_TREES then
call SetRect(.Rect1,newx1 - radius,newy1 - radius,newx1 + radius,newy1 + radius)
call EnumDestructablesInRect(.Rect1,.Tree_filt,function Data.destroyenumtrees)
endif
set flame = CreateUnit(GetOwningPlayer(d.cast),UNIT_ID,newx2,newy2,0.00)
call SetUnitScale(flame,(SCALE - Scale_reduction(d.spread)),(SCALE - Scale_reduction(d.spread)),0.00)
call DestroyEffect(AddSpecialEffect(BEGIN_SFX,newx2,newy2))
call UnitApplyTimedLife(flame,039;BTLF039;,Duration(d.level))
call SetUnitAbilityLevel(flame,ABIL2_ID,d.level)
call PauseUnit(flame,true)
call PauseUnit(flame,false)
call GroupEnumUnitsInRange(.Group,newx2,newy2,radius,.Enemy_filt)
loop
set first = FirstOfGroup(.Group)
exitwhen first == null
call GroupRemoveUnit(.Group,first)
if IsUnitEnemy(first,GetOwningPlayer(d.cast)) then
call UnitDamageTarget(d.cast,first,Damage(d.level),false,false,A_TYPE,D_TYPE,W_TYPE)
call DestroyEffect(AddSpecialEffectTarget(DAMAGE_SFX,first,DAMAGE_POINT))
endif
endloop
if DESTROY_TREES then
call SetRect(.Rect1,newx2 - radius,newy2 - radius,newx2 + radius,newy2 + radius)
call EnumDestructablesInRect(.Rect1,.Tree_filt,function Data.destroyenumtrees)
endif
endif
set i = i + 1
endloop
if .DT <= 0 then
call PauseTimer(.Timer)
set .DT = 0
endif
set flame = null
set first = null
endmethod
static method actions takes nothing returns boolean
local Data d = Data.create()
local location l = GetSpellTargetLoc()
local unit flame = null
local unit first = null
local real angle = 0.00
set d.cast = GetTriggerUnit()
set d.castx = GetUnitX(d.cast)
set d.casty = GetUnitY(d.cast)
set d.locx = .safex(GetLocationX(l))
set d.locy = .safey(GetLocationY(l))
set angle = Atan2((d.locy - d.casty),(d.locx - d.castx))
set d.sin1 = Sin(angle + (90.00 * bj_DEGTORAD))
set d.sin2 = Sin(angle - (90.00 * bj_DEGTORAD))
set d.cos1 = Cos(angle + (90.00 * bj_DEGTORAD))
set d.cos2 = Cos(angle - (90.00 * bj_DEGTORAD))
set d.level = GetUnitAbilityLevel(d.cast,ABIL_ID)
set d.spread = 0
set flame = CreateUnit(GetOwningPlayer(d.cast),UNIT_ID,d.locx,d.locy,0.00)
call SetUnitScale(flame,SCALE,SCALE,0.00)
call DestroyEffect(AddSpecialEffect(BEGIN_SFX,d.locx,d.locy))
call UnitApplyTimedLife(flame,039;BTLF039;,Duration(d.level))
call SetUnitAbilityLevel(flame,ABIL2_ID,d.level)
call PauseUnit(flame,true)
call PauseUnit(flame,false)
call GroupEnumUnitsInRange(.Group,d.locx,d.locy,Radius(d.level),.Enemy_filt)
loop
set first = FirstOfGroup(.Group)
exitwhen first == null
call GroupRemoveUnit(.Group,first)
if IsUnitEnemy(first,GetOwningPlayer(d.cast)) then
call UnitDamageTarget(d.cast,first,Damage(d.level),false,false,A_TYPE,D_TYPE,W_TYPE)
call DestroyEffect(AddSpecialEffectTarget(DAMAGE_SFX,first,DAMAGE_POINT))
endif
endloop
if DESTROY_TREES then
call SetRect(.Rect1,d.locx - Radius(d.level),d.locy - Radius(d.level),d.locx + Radius(d.level),d.locy + Radius(d.level))
call EnumDestructablesInRect(.Rect1,.Tree_filt,function Data.destroyenumtrees)
endif
set .DT = .DT + 1
set .D[.DT] = d
if .DT == 1 then
call TimerStart(.Timer,INTERVAL,true,function Data.update)
endif
call RemoveLocation(l)
set flame = null
set first = null
set l = null
return false
endmethod
static method onInit takes nothing returns nothing
set .Timer = CreateTimer()
set .Group = CreateGroup()
set .Rect1 = Rect(0.00,0.00,1.00,1.00)
set .Tree_filt = Filter(function Data.treefilt)
set .Enemy_filt = Filter(function Data.enemyfilt)
set .Game_maxX = GetRectMaxX(bj_mapInitialPlayableArea) - 64.00
set .Game_maxY = GetRectMaxY(bj_mapInitialPlayableArea) - 64.00
set .Game_minX = GetRectMinX(bj_mapInitialPlayableArea) + 64.00
set .Game_minY = GetRectMinY(bj_mapInitialPlayableArea) + 64.00
// Register event.
call GT_AddStartsEffectAction(function Data.actions,ABIL_ID)
set .Tree_dummy = CreateUnit(Player(PLAYER_NEUTRAL_PASSIVE),TREEDUMMY_ID,0.00,0.00,0.00)
call SetUnitPathing(.Tree_dummy,false)
call ShowUnit(.Tree_dummy,false)
if ALLOW_PRELOAD then
call DestroyEffect(AddSpecialEffect(BEGIN_SFX,0.00,0.00))
call DestroyEffect(AddSpecialEffect(DAMAGE_SFX,0.00,0.00))
endif
endmethod
endstruct
endscope
</i></i>
Sanity's Eclipse
JASS?: Yes, vJass.
JESP?: Yes.
Leakless?: Yes.
Lagless?: Yes.
MUI?: Yes.
Requires: NewGen and GTrigger.
Description:
Found in my next post (Post #4).
Screenshot:
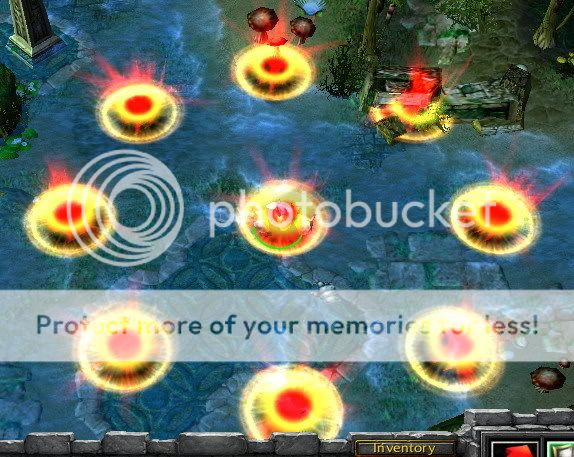
Script:
JASS:
// Found in my next post (Post #4).
Change log:
Found in my next post (Post #4).