Infinitegde
O.O
- Reaction score
- 86
Hell's Cage
By Infinitegde
Note: Although I like to make compact spells, this is the first time I've made so many triggers for one spell
... Anyway, the main purpose of this spell was to show people how amazing Spherical Coordinates are. I was able to create 7 Unique movement patterns by altering just the STARTING PLACES of the objects. If one was to change the rate of increase and all that, the variations could be limitless. Also, to be exact I only changed one VARIABLE that is involved in making the starting places
Thats how many variations there are.
Description: Single Target Spell. Cast on an enemy unit, pulls the unit into the air, creates numerous amounts of fireballs around the unit, and entraps it. Enclosing it until it takes up no space then forcefully ripping it apart.
Screenshot:
-Notice, casting spell on two different targets at the same time! MUI!
What Happens: Lifts Target up, fire balls encircle it, unit gets smaller as fireballs get closer, deals damage as unit hits ground.
MUI: Yes, can only cast one instance of the spell on the same unit though.
Coded In: vJass
Variable Locations:
Ability codes and Unit codes are in globals block in the beggining of map, other variables are in the struct, "HellsCage"
Limitations:
-None, suggest you make max amount of fireballs at 30-40. More will cause a LOT of lag. But my computer sucks, so it might not lag your computers...
Features:
-Spherical Coordinates! YAY!
-A LOT of variations, only 7 included, but there are many more, will experiment and make more.
-A lot of changable variables
Implementation:
1) Import ability Hells Cage and unit Fireball.
2) Copy and Paste the code in map Header into your map.
3) Create 6 triggers named as the triggers in this map. (Case Sensitive)
4) Copy and Paste code from each trigger into each trigger respectively.
5) Change ability codes and unit codes to the respective codes in you map. (Press Ctrl+D in the object editor to see codes)
6) Change variables in the map header as you like and your ready to go.
Map Header Code:
Trigger 1: Start
Trigger 2:Rise
Trigger 3: Create Cage
Trigger 4:Rotation
Trigger 5: Explosion
Trigger 6: Clean Up
By Infinitegde
Note: Although I like to make compact spells, this is the first time I've made so many triggers for one spell
Description: Single Target Spell. Cast on an enemy unit, pulls the unit into the air, creates numerous amounts of fireballs around the unit, and entraps it. Enclosing it until it takes up no space then forcefully ripping it apart.
Screenshot:
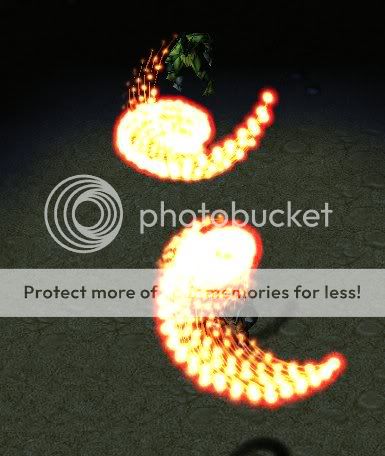
-Notice, casting spell on two different targets at the same time! MUI!
What Happens: Lifts Target up, fire balls encircle it, unit gets smaller as fireballs get closer, deals damage as unit hits ground.
MUI: Yes, can only cast one instance of the spell on the same unit though.
Coded In: vJass
Variable Locations:
Ability codes and Unit codes are in globals block in the beggining of map, other variables are in the struct, "HellsCage"
Limitations:
-None, suggest you make max amount of fireballs at 30-40. More will cause a LOT of lag. But my computer sucks, so it might not lag your computers...
Features:
-Spherical Coordinates! YAY!
-A LOT of variations, only 7 included, but there are many more, will experiment and make more.
-A lot of changable variables
Implementation:
1) Import ability Hells Cage and unit Fireball.
2) Copy and Paste the code in map Header into your map.
3) Create 6 triggers named as the triggers in this map. (Case Sensitive)
4) Copy and Paste code from each trigger into each trigger respectively.
5) Change ability codes and unit codes to the respective codes in you map. (Press Ctrl+D in the object editor to see codes)
6) Change variables in the map header as you like and your ready to go.
Map Header Code:
JASS:
globals
//Ability code: Change it to the Abil Code of Hell's Cage
integer HellC = 039;A002039;
integer Invul = 039;Avul039;
integer Crow = 039;Amrf039;
//Unit Code: Fireballs unit Code
integer FireC = 039;u000039;
//Hells Cage Variables
group HellsCageTarget = CreateGroup()
endglobals
struct HellsCage
//Change these:
integer MovementStyle = 3
//Set this to 0 for Erratic movement.
//1 for Snake like movement.
//2 for Ordered-1 movement
//3 for Ordered-2 Movement. <<Tis cooler than 2 <img src="data:image/gif;base64,R0lGODlhAQABAIAAAAAAAP///yH5BAEAAAAALAAAAAABAAEAAAIBRAA7" class="smilie smilie--sprite smilie--sprite8" alt=":D" title="Big Grin :D" loading="lazy" data-shortname=":D" />
//4 for Sin Transformation movement.
//5 for Tan Transformation movement. (May not look ordered, but look at shadows!)
// Num of Fireballs per level
integer NumofFireballs = 10
real DamagePerFire = 10.00
//Circle Radius, SHOULD BE GREATER THAN 135!
real rho = 150.00
//At what height should the fireballs appear?
real FHeight = 500.00
//Don't Change
unit Targ
unit Cast
integer Lvl
group Fire = CreateGroup()
integer Stage
endstruct
struct FireLoc
unit Targ
unit Cast
real Deg
real ZDeg
real Radius
real Scale = 1.00
integer count = 0
endstruct
Trigger 1: Start
JASS:
function Trig_Start_Conditions takes nothing returns boolean
if ( not ( GetSpellAbilityId() == HellC ) ) then
return false
endif
return true
endfunction
function Trig_Start_Actions takes nothing returns nothing
local effect spec
local unit Caster
local unit Target
local location specloc
local HellsCage new = HellsCage.create()
set Caster = GetTriggerUnit()
set Target = GetSpellTargetUnit()
call PauseUnit(Target, true)
set new.Targ = Target
set specloc = GetUnitLoc(Target)
set spec = AddSpecialEffectLoc("Abilities\\Spells\\Human\\Resurrect\\ResurrectTarget.mdl", specloc)
call DestroyEffect(spec)
call RemoveLocation(specloc)
set new.Stage = 0
set new.Lvl = GetUnitAbilityLevel(Caster,HellC)
set new.Cast = Caster
call UnitAddAbility(Target,Crow)
call IssueImmediateOrder( Caster, "stop" )
call PauseUnit(Caster, true)
call SetUnitAnimation( Caster, "spell" )
call AddUnitAnimationProperties(Caster, "loop", true )
call UnitAddAbility(Caster,Invul)
call UnitAddAbility(Target,Invul)
call SetUnitUserData(Target,new)
call GroupAddUnit(HellsCageTarget, Target)
endfunction
//===========================================================================
function InitTrig_Start takes nothing returns nothing
set gg_trg_Start = CreateTrigger( )
call TriggerRegisterAnyUnitEventBJ( gg_trg_Start, EVENT_PLAYER_UNIT_SPELL_EFFECT )
call TriggerAddCondition( gg_trg_Start, Condition( function Trig_Start_Conditions ) )
call TriggerAddAction( gg_trg_Start, function Trig_Start_Actions )
endfunction
Trigger 2:Rise
JASS:
function Rise_Actions takes nothing returns nothing
local HellsCage new = GetUnitUserData(GetEnumUnit())
local unit Target = new.Targ
if(new.Stage == 0)then
call SetUnitFlyHeightBJ(Target, GetUnitFlyHeight(Target)+10.00,1000)
if(GetUnitFlyHeight(Target)>new.FHeight) then
set new.Stage = 1
call ConditionalTriggerExecute( gg_trg_Create_Cage )
endif
endif
set Target = null
endfunction
function Trig_Rise_Actions takes nothing returns nothing
call ForGroup(HellsCageTarget,function Rise_Actions)
endfunction
//===========================================================================
function InitTrig_Rise takes nothing returns nothing
set gg_trg_Rise = CreateTrigger( )
call TriggerRegisterTimerEventPeriodic( gg_trg_Rise, 0.01 )
call TriggerAddAction( gg_trg_Rise, function Trig_Rise_Actions )
endfunction
Trigger 3: Create Cage
JASS:
function Create_Cage takes nothing returns nothing
local HellsCage new = GetUnitUserData(GetEnumUnit())
local FireLoc Test
local effect spec
local integer count = 0
local location polarloc
local location temp
local unit Target = new.Targ
local unit u
local location specloc
local integer Num = new.Lvl*new.NumofFireballs
local integer Factor = 360/Num
local integer MovementStyle = new.MovementStyle
if(new.Stage == 1) then
loop
exitwhen count == Num
set Test = FireLoc.create()
set temp = GetUnitLoc(Target)
set Test.Deg = (count*Factor)
set Test.Radius = new.rho
if(MovementStyle == 0) then
set Test.ZDeg = count*count*5
endif
if(MovementStyle == 1) then
set Test.ZDeg = 10*count
endif
if(MovementStyle == 2) then
set Test.ZDeg = 90
endif
if(MovementStyle == 3) then
set Test.ZDeg = SquareRoot(Test.Deg*count)
endif
if(MovementStyle == 4) then
set Test.ZDeg = Sin(Test.Deg*bj_DEGTORAD)*100
endif
if(MovementStyle == 5) then
set Test.ZDeg = Tan(Test.Deg*bj_DEGTORAD)*100
endif
set polarloc = Location(GetLocationX(temp) + new.rho * Cos((count*Factor) * bj_DEGTORAD),GetLocationY(temp) + new.rho * Sin((count*Factor) * bj_DEGTORAD))
set u = CreateUnitAtLoc(GetOwningPlayer(new.Cast),FireC,polarloc,0)
set Test.Targ = Target
set Test.Cast = new.Cast
call GroupAddUnit(new.Fire,u)
call SetUnitFlyHeight(u, GetUnitFlyHeight(Target), 10000)
call SetUnitUserData(u,Test)
set u = null
call RemoveLocation(temp)
call RemoveLocation(polarloc)
set count = count+1
endloop
set specloc = GetUnitLoc(Target)
set spec = AddSpecialEffectLoc("Abilities\\Spells\\Human\\Resurrect\\ResurrectTarget.mdl", specloc)
call DestroyEffect(spec)
call RemoveLocation(specloc)
set new.Stage = 2
endif
set Target = null
endfunction
function Trig_Create_Cage_Actions takes nothing returns nothing
call ForGroup(HellsCageTarget,function Create_Cage)
endfunction
//===========================================================================
function InitTrig_Create_Cage takes nothing returns nothing
set gg_trg_Create_Cage = CreateTrigger( )
call TriggerAddAction( gg_trg_Create_Cage, function Trig_Create_Cage_Actions )
endfunction
Trigger 4:Rotation
JASS:
function Rotate takes nothing returns nothing
local unit u = GetEnumUnit()
local FireLoc new = GetUnitUserData(u)
local unit Target = new.Targ
local HellsCage TEST = GetUnitUserData(Target)
local real X = GetUnitX(Target)
local real Y = GetUnitY(Target)
local real Z = GetUnitFlyHeight(Target)
local real NDeg
local real NZDeg
local real radius = new.Radius
set new.Radius = new.Radius - 0.20
set new.Deg = new.Deg + 3
set new.ZDeg = new.ZDeg + 3
set NDeg = new.Deg
set NZDeg = new.ZDeg
if(radius < 135) then
call SetUnitScale(Target,new.Scale,new.Scale,new.Scale)
set new.count = new.count+1
if (new.Scale > 0) and new.count > 5 then
set new.Scale = new.Scale - 0.01
set new.count = 0
endif
endif
set X = X + radius*Sin(NZDeg*bj_DEGTORAD)*Cos(NDeg*bj_DEGTORAD)
set Y = Y + radius*Sin(NZDeg*bj_DEGTORAD)*Sin(NDeg*bj_DEGTORAD)
set Z = Z + radius*Cos(NZDeg*bj_DEGTORAD)
call SetUnitX(u,X)
call SetUnitY(u,Y)
call SetUnitFlyHeight(u,Z,0)
if (radius <1) then
set TEST.Stage = 3
call ConditionalTriggerExecute(gg_trg_Explosion)
endif
endfunction
function RotateShell takes nothing returns nothing
local HellsCage new = GetUnitUserData(GetEnumUnit())
if (new.Stage == 2)then
call ForGroup(new.Fire,function Rotate)
endif
endfunction
function Trig_Rotation_Actions takes nothing returns nothing
call ForGroup(HellsCageTarget,function RotateShell)
endfunction
//===========================================================================
function InitTrig_Rotation takes nothing returns nothing
set gg_trg_Rotation = CreateTrigger( )
call TriggerRegisterTimerEventPeriodic( gg_trg_Rotation, 0.03 )
call TriggerAddAction( gg_trg_Rotation, function Trig_Rotation_Actions )
endfunction
Trigger 5: Explosion
JASS:
function Explode takes nothing returns nothing
local HellsCage new = GetUnitUserData(GetEnumUnit())
local unit Target = new.Targ
local unit Caster = new.Cast
local location pos = GetUnitLoc(Target)
local effect spec
if(new.Stage==3)then
call SetUnitScale(Target,1.00,1.00,1.00)
set spec = AddSpecialEffectLoc("Abilities\\Spells\\Items\\TomeOfRetraining\\TomeOfRetrainingCaster.mdl", pos )
call DestroyEffect(spec)
call PauseUnit(Target, false)
call PauseUnit(Caster,false)
call AddUnitAnimationProperties(Caster, "loop", false )
call SetUnitFlyHeight(Target,0,0)
call UnitRemoveAbility(Target,Crow)
call UnitRemoveAbility(Caster,Invul)
call UnitRemoveAbility(Target,Invul)
set new.Stage = 4
endif
set Caster = null
set Target = null
call RemoveLocation(pos)
endfunction
function Trig_Explosion_Actions takes nothing returns nothing
call ForGroup(HellsCageTarget,function Explode)
call TriggerSleepAction(0.01)
call ConditionalTriggerExecute(gg_trg_Clean_Up)
endfunction
//===========================================================================
function InitTrig_Explosion takes nothing returns nothing
set gg_trg_Explosion = CreateTrigger( )
call TriggerAddAction( gg_trg_Explosion, function Trig_Explosion_Actions )
endfunction
Trigger 6: Clean Up
JASS:
function Remove takes nothing returns nothing
local unit u = GetEnumUnit()
local FireLoc Rem = GetUnitUserData(u)
local HellsCage test = GetUnitUserData(Rem.Targ)
local real dmg = test.DamagePerFire
set Rem = GetUnitUserData(u)
call UnitDamageTarget(test.Cast,test.Targ, dmg, true, false, ATTACK_TYPE_CHAOS, DAMAGE_TYPE_FIRE,WEAPON_TYPE_WHOKNOWS)
call Rem.destroy()
call GroupRemoveUnit(test.Fire,u)
call RemoveUnit(u)
set u = null
endfunction
function CleanUp takes nothing returns nothing
local HellsCage new = GetUnitUserData(GetEnumUnit())
if(new.Stage == 4) then
call ForGroup(new.Fire,function Remove)
call GroupRemoveUnit(HellsCageTarget,new.Targ)
call new.destroy()
endif
endfunction
function Trig_Untitled_Trigger_001_Actions takes nothing returns nothing
call ForGroup(HellsCageTarget,function CleanUp)
endfunction
//===========================================================================
function InitTrig_Clean_Up takes nothing returns nothing
set gg_trg_Clean_Up = CreateTrigger( )
call TriggerAddAction( gg_trg_Clean_Up, function Trig_Untitled_Trigger_001_Actions )
endfunction