Knockback System (KBS)
by kenny!
v2.04
by kenny!
v2.04
Basically, I'm submitting this system because I think it is worthy. However, for all those people who wonder why we need another knockback system, here are some other reasons:
- This system is fully documented and easy to use.
- Runs on one timer. I believe the only other systems to do this are emjlr3's and uberplayer's (on this site).
- Has easily changeable special effects.
- It is also completely MUI and needs no other systems at all.
So, I originally made this system well over a year ago and it leaked and was slow etc etc. So a few months ago i ported it to VJASS to fix it up a bit, finding out that i still had a handle leak problem which i could not for the life of me solve. Then i tried again a little while ago and fixed it. So i put some documentation in and got it ready to submit.
I tried to make the system easy enough for GUI users to use, as well as JASS users. Its simplistic form allows for basic uses and more creative users for those more experienced.
Heres the code:
JASS:
library KBS initializer Init
//***************************************************************************************
//** **
//** Knockback System (KBS) **By kenny!** **
//** v 2.04 **
//** **
//** A system I made that can be used for knockback spells in a variety of **
//** applications in a map. User defined variables make it easy to give the desired **
//** effect for any spell. **
//** **
//** Requires: **
//** - A vJASS preprocessor (NewGen Pack). **
//** - No other systems. **
//** - A dummy unit. One can be found in this map ('u000') **
//** - The special effects found in the import/export section of this map. **
//** They are the "Dust.mdx" and the "SlideWater.mdx". Or you can use your **
//** own if you have some. **
//** **
//***************************************************************************************
//***************************************************************************************
//** **
//** Other Information: **
//** - Angle is taken in degrees. But can easily be changed if you know how. **
//** - Units will be stopped if they hit the map borders. **
//** - Remember to import the special effect into your map if you need them. **
//** - There are two functions that can be used in this system. **
//** - NEW FUNCTION: KBS_BeginCommon() is for all the common knockback uses in **
//** your map. It only takes 4 parameters, and use global parameters for Area **
//** AllowMove and CheckPathing. Making it an icredibly easy function to use. **
//** **
//** Sliding Distances: **
//** - This is some general knowledge on the different distances and how to **
//** get them. **
//** - Sliding distances have changed in this version of the system. **
//** - The function call (KBS_Begin/Ex) now takes distance and duration **
//** parameters instead of startspeed and deceleration. **
//** - Therefore the parameter 'Distance' will set the length of the knockback **
//** while the parameter 'Duration" will set the time it will take. **
//** **
//***************************************************************************************
//***************************************************************************************
//** **
//** Implementation: **
//** - First off you need the NewGen world editor. This is a must. **
//** - Copy this trigger into your map. Or make a trigger in your map and **
//** convert it to custom text. Then copy this text into that trigger. **
//** - Now you will either need to export the special effects from this map to **
//** your map, or you will need your own. I do not take credit for the **
//** special effects found in this map. **
//** - Once you have the effects in your map. Find the configuration block **
//** that is underneath all this green text. Change the WATER_SFX and the **
//** DIRT_SFX strings to the ones in your map. These should be the same if **
//** you exported the ones from this map. **
//** - Make sure you have the dummy unit needed. It can be any unit, but i **
//** suggest you use the one found in this map, it is labelled 'Dummy Unit' **
//** or 'u000'. **
//** - Your done! Save your map, make a spell using this system and try it out. **
//** **
//** NOTE: Please report any bugs to me at thehelper.net via PM. (User name: kenny!)**
//** **
//***************************************************************************************
//***************************************************************************************
//** **
//** Usage: **
//** **
//** call KBS_Begin(Target,Distance,Duration,Angle,Area) **
//** or **
//**call KBS_BeginEx(Target,Distance,Duration,Angle,Effect,Area,AllowMove,CheckPathing)**
//** or **
//** call KBS_BeginCommon(Target,Distance,Duration,Angle) **
//** **
//** - Target - The unit that will be used in the knockback. **
//** - Distance - The distance of the knockback (how far the unit will slide). **
//** - Duration - The duration of the knockback refers to how long the unit **
//** will slide for. **
//** - Angle - The angle that the unit will be knocked back at. **
//** THIS MUST BE IN DEGREES. <- Easier for GUI users. **
//** - Effect - This is only used in the extended function. If you do not **
//** want one of the predefined effects, you can choose your own. **
//** However, this effect is attached to the unit and removed at **
//** the end, so non-looping or repeating effect wont work. **
//** - Area - This determines whether or not trees will be knocked down. **
//** For trees to be knocked down, a positive number (real) must **
//** be used, such as 150.00, which would give a radius of 150.00 **
//** in which trees will be knocked down. **
//** For trees to not be knocked down, a negative number (real) **
//** must be used, such as -150.00, which would create a radius **
//** that if a tree comes within it, the unit will stop moving. **
//** For none of those effects, the number 0 (0.00) can be used. **
//** This will just cause the units to "bounce" off trees. **
//** -Allowmove - This boolean will decided whether or not you want the unit **
//** to have the ability to move while being knocked back. **
//** "true" will allow them to move, while "false" will not. **
//** -CheckPathing - A boolean that, if true, will check for unpathable terrain **
//** such as a wall or cliff, or where doodads may be. If false **
//** it will ignore these changes and the unit will be pushed **
//** along the wall, cliff or doodad. **
//** **
//** REMEMBER: Positive = trees destroyed, Negative = trees not destroyed. **
//** **
//** Example of Usage: **
//** **
//** -call KBS_Begin(target,500.00,2.00,270.00,150.00) **
//** or **
//** -call KBS_BeginEx(target,500.00,2.00,270.00,"Effect.mdl",-150.00,true,true) **
//** **
//** The first one will cause the target unit of a spell to be knocked back 500.00 **
//** range over a 2.00 second duration. It will be knocked back at an angle of **
//** 270.00, which I'm pretty sure is south, and it will knock down trees within **
//** a 150.00 radius around the unit. **
//** **
//** The bottom one of the two will do the exact same as the top, however you are **
//** able to choose your own special effect that will be attached to the unit. As **
//** you can see, the number for Area (destroying trees) is negative, therefore **
//** no trees will be knocked down, and the unit will stop moving if it comes in **
//** contact with a tree. There is also an extra boolean nearing end, this is for **
//** allowing units to move while sliding. It is true, meaning units are allowed. **
//** There is also an added feature that will check for pathable terrain and stop a **
//** unit if it comes in contact with things such as walls, cliffs or doodads. **
//** If true, the unit will stop moving if it comes in direct contact will a wall **
//** or cliff. **
//** **
//***************************************************************************************
//***************************************************************************************
//** **
//** Other functions: **
//** **
//** - call KBS_IsUnitSliding(Target) **
//** **
//** - This function checks if a picked unit is currently sliding using this **
//** system. It will return true if it is. **
//** **
//** - call KBS_StopUnitSliding(Target) **
//** **
//** - This function will stop a picked unit from sliding (using this system). **
//** It also returns true if the unit is stopped. **
//** **
//** - These functions can be used in conjunction with each other, by checking if a **
//** unit is sliding then stopping it if it is. The regular Storm Bolt spell in **
//** the Test Spells section uses these two function in conjunction with each **
//** other as an example. **
//** **
//***************************************************************************************
//***************************************************************************************
//** **
//** Some points on checking for pathable terrain: **
//** **
//**` - The area specified for destroying trees must be at least 75.00-100.00 range **
//** larger than the distance for checking for pathing, which is below in the **
//** globals block. **
//** - When using KBS_BeginEx() and using either 0.00 or a negative value for the **
//** parameter 'Area' (destroying trees) it is better to have CheckPathing as **
//** FALSE. This is due to the fact that setting CheckPathing to TRUE will **
//** override the 'Area' Parameter and stop a unit if it gets near trees. **
//** - Basically what this means is that you cannot get a unit to just 'bounce' off **
//** trees anymore, it is either destroy trees or get stopped by them. **
//** **
//***************************************************************************************
globals
// CONFIGURABLES:
private constant integer DUMMY_ID = 039;u000039; // This is the dummy unit.
private constant real INTERVAL = 0.04 // Recommended Interval.
private constant string ATTACH_POINT = "origin" // Attachment point for effects.
private constant string WATER_SFX = "SlideWater.mdx" // Water slide effect.
private constant string DIRT_SFX = "Dust.mdx" // Ground slide effect.
private constant string COLLISION_SFX = "Abilities\\Weapons\\AncientProtectorMissile\\AncientProtectorMissile.mdl" // Collision effect when unit hits a wall/cliff etc.
private constant boolean SHOW_COLLISION = true // Whether or not effect will show if a unit collides with a wall/cliff etc.
// The follow are used when using KBS_BeginCommon() for the common parameters that will be used.
private constant real COMMON_AREA = 150.00 // The area in which trees will be destroyed.
private constant boolean COMMON_ALLOW_MOVE = true // Whether or not to allow units to move.
private constant boolean COMMON_CHECK_PATHING = true // Whether or not to check pathing.
// The following raw codes will probably not need to be changed for most maps.
private constant integer HARVEST = 039;Ahrl039; // Raw code for: Harvest(Ghouls Lumber).
private constant integer LOCUST = 039;Aloc039; // Raw code for Locust ability.
// The following value is used for terrain pathability checking.
private constant real DISTANCE = 50.00
// The above is the distance in front of the unit that terrain pathability
// will be checked. A value of 40.00-50.00 is recommended. Also make sure
// that this distance is AT LEAST 75.00-100.00 units less than the ranges set
// for destroying trees, otherwise it wont work properly.
// Example: Keep above distance at 50.00, and use 150.00 for knocking down trees.
// This seems to work very well.
private constant real MAX_RANGE = 10.00
// Max range for checking for pathability. I suggest you leave this alone.
endglobals
//***************************************************************************************
//** **
//** DO NOT TOUCH BELOW HERE UNLESS YOU KNOW WHAT YOUR ARE DOING! **
//** **
//***************************************************************************************
private keyword Data
globals
private Data array D
private boolean array BA
private item array Hidden
private integer Hidden_max = 0
private integer Total = 0
private real Game_maxX = 0.00
private real Game_minX = 0.00
private real Game_maxY = 0.00
private real Game_minY = 0.00
private rect Rect1 = null
private unit Tree_dummy = null
private item Item = null
private timer Timer = null
private boolexpr Tree_filt = null
endglobals
//=======================================================================
private function Filter_items takes nothing returns nothing
if IsItemVisible(GetEnumItem()) then
set Hidden[Hidden_max] = GetEnumItem()
call SetItemVisible(Hidden[Hidden_max],false)
set Hidden_max = Hidden_max + 1
endif
endfunction
// Thanks to Vexorian for original concept, and Anitarf for the up-to-date version. (Slightly midified).
private function Check_pathability takes real x1, real y1 returns boolean
local real x2 = 0.00
local real y2 = 0.00
call SetRect(Rect1,0.00,0.00,128.00,128.00)
call MoveRectTo(Rect1,x1,y1)
call EnumItemsInRect(Rect1,null,function Filter_items)
call SetItemPosition(Item,x1,y1)
set x2 = GetItemX(Item)
set y2 = GetItemY(Item)
call SetItemVisible(Item,false)
loop
exitwhen Hidden_max <= 0
set Hidden_max = Hidden_max - 1
call SetItemVisible(Hidden[Hidden_max],true)
set Hidden[Hidden_max] = null
endloop
return (x1 - x2) * (x1 - x2) + (y1 - y2) * (y1 - y2) < MAX_RANGE * MAX_RANGE
endfunction
//=======================================================================
private function Destroy_trees takes nothing returns nothing
if BA[0] then
call KillDestructable(GetEnumDestructable()) // Used to destroy destructables.
else
set BA[1] = true
endif
endfunction
// Thanks to PitzerMike for this function. (Modified Slightly).
private function Check_trees takes nothing returns boolean
local destructable dest = GetFilterDestructable()
local boolean result = false
if GetDestructableLife(dest) > 0.405 then
call ShowUnit(Tree_dummy,true)
call SetUnitX(Tree_dummy,GetWidgetX(dest))
call SetUnitY(Tree_dummy,GetWidgetY(dest))
set result = IssueTargetOrder(Tree_dummy,"harvest",dest)
call IssueImmediateOrder(Tree_dummy,"stop")
call ShowUnit(Tree_dummy,false)
set dest = null
return result
endif
set dest = null
return result
endfunction
// Checks if a point is on the map boundaries.
private function Outside_bounds takes real x, real y returns boolean
return (x > Game_maxX or y > Game_maxY or x < Game_minX or y < Game_minY)
endfunction
//=======================================================================
private struct Data
unit targ = null
real speed = 0.00
real decrease = 0.00
real sin = 0.00
real cos = 0.00
real radius = 0.00
integer sfxmode = 0
effect sfx = null
boolean custom = false
boolean allowmove = false
boolean pathing = false
boolean forcestop = false
// Checking for terrain type.
method terrain takes nothing returns integer
local real x = GetUnitX(.targ)
local real y = GetUnitY(.targ)
if IsTerrainPathable(x,y,PATHING_TYPE_FLOATABILITY) then
return 1
elseif not IsTerrainPathable(x,y,PATHING_TYPE_WALKABILITY) then
return 2
endif
return 0
endmethod
static method create takes unit Target, real Distance, real Duration, real Angle, string Effect, real Area, boolean AllowMove, boolean CheckPathing returns Data
local Data d = Data.allocate()
// Allocates struct members to user defined variables.
set d.targ = Target
set d.speed = (2.00 * Distance) / (Duration + 1.00)
set d.decrease = d.speed / Duration
set d.sin = Sin(Angle)
set d.cos = Cos(Angle)
set d.radius = Area
set d.allowmove = AllowMove
set d.pathing = CheckPathing
set d.sfxmode = d.terrain()
if Effect != "" and Effect != null then
set d.custom = true
endif
// Adding effects to the unit.
if d.custom then
set d.sfx = AddSpecialEffectTarget(Effect,d.targ,ATTACH_POINT)
else
if d.sfxmode == 1 then
set d.sfx = AddSpecialEffectTarget(DIRT_SFX,d.targ,ATTACH_POINT)
elseif d.sfxmode == 2 then
set d.sfx = AddSpecialEffectTarget(WATER_SFX,d.targ,ATTACH_POINT)
endif
endif
return d
endmethod
private method onDestroy takes nothing returns nothing
set .targ = null
if .sfx != null then
call DestroyEffect(.sfx) // Destroys effects if needed.
set .sfx = null
endif
set BA[0] = false
set BA[1] = false
endmethod
endstruct
//=======================================================================
private function Update takes nothing returns nothing
local integer i = 1
local integer newmode = 0
local integer height = 0
local Data d = 0
local real x = 0.00
local real y = 0.00
local real newx = 0.00
local real newy = 0.00
loop
exitwhen i > Total
set d = D<i>
set newmode = d.sfxmode
set x = GetUnitX(d.targ)
set y = GetUnitY(d.targ)
// Destroys trees if wanted, or stops the unit
if d.radius != 0.00 then
set BA[0] = d.radius > 0.00
call SetRect(Rect1,x - d.radius,y - d.radius,x + d.radius,y + d.radius)
call EnumDestructablesInRect(Rect1,Tree_filt,function Destroy_trees)
endif
// Checks for terrain pathability, such as walls and cliffs.
if d.pathing then
if Check_pathability(x + DISTANCE * d.cos,y + DISTANCE * d.sin) == false then
set height = 1
if SHOW_COLLISION then
call DestroyEffect(AddSpecialEffect(COLLISION_SFX,x,y))
endif
endif
endif
if not d.custom then
set d.sfxmode = d.terrain() // Checks for pathing again.
// Adds special effect if terrain changes.
if d.sfxmode == 1 and newmode == 2 then
call DestroyEffect(d.sfx)
set d.sfx = AddSpecialEffectTarget(DIRT_SFX,d.targ,ATTACH_POINT)
elseif d.sfxmode == 2 and newmode == 1 then
call DestroyEffect(d.sfx)
set d.sfx = AddSpecialEffectTarget(WATER_SFX,d.targ,ATTACH_POINT)
endif
endif
if d.speed <= 0 or Outside_bounds(x,y) or BA[1] or height == 1 or d.forcestop == true then
call d.destroy() // Finish knockback
set D<i> = D[Total]
set Total = Total - 1
set i = i - 1
set height = 0
else
set newx = x + d.speed * d.cos
set newy = y + d.speed * d.sin
// Allows unit to move while sliding, if specified.
if d.allowmove then
call SetUnitX(d.targ,newx)
call SetUnitY(d.targ,newy)
else
call SetUnitPosition(d.targ,newx,newy)
endif
set d.speed = d.speed - d.decrease // Sets new speed.
endif
set i = i + 1
endloop
if Total <= 0 then
call PauseTimer(Timer)
set Total = 0
endif
endfunction
//=======================================================================
// Checks if a unit is sliding - returns true if it is.
public function IsUnitSliding takes unit Target returns boolean
local integer i = 1
loop
exitwhen i > Total
if D<i>.targ == Target then
return true
endif
set i = i + 1
endloop
return false
endfunction
//=======================================================================
// Stops a unit from sliding - returns true if a unit is stopped.
public function StopUnitSliding takes unit Target returns boolean
local integer i = 1
loop
exitwhen i > Total
if D<i>.targ == Target then
set D<i>.forcestop = true
return true
endif
set i = i + 1
endloop
return false
endfunction
//=======================================================================
// Extended function - gives the most customisation for a single unit knockback.
public function BeginEx takes unit Target, real Distance, real Duration, real Angle, string Effect, real Area, boolean AllowMove, boolean CheckPathing returns boolean
local Data d = 0
if Target == null or Distance <= 0.00 or Duration <= 0.00 then
debug call BJDebugMsg("Error: Invalid input in KBS_Begin(Ex)") // Error message.
return false
endif
set d = Data.create(Target,Distance,(Duration/INTERVAL),(Angle * 0.01745328),Effect,Area,AllowMove,CheckPathing)
set Total = Total + 1
if Total == 1 then
call TimerStart(Timer,INTERVAL,true,function Update)
endif
set D[Total] = d
return true
endfunction
//=======================================================================
// Basic function - Can be used in a wide variety of ways and gives a basic knockback.
public function Begin takes unit Target, real Distance, real Duration, real Angle, real Area returns nothing
call BeginEx(Target,Distance,Duration,Angle,"",Area,false,false)
endfunction
// Common function - For all basic knockback needs, easy to use and simple to remember.
public function BeginCommon takes unit Target, real Distance, real Duration, real Angle returns nothing
call BeginEx(Target,Distance,Duration,Angle,null,COMMON_AREA,COMMON_ALLOW_MOVE,COMMON_CHECK_PATHING)
endfunction
//=======================================================================
// Sets map boundries and sets timer, rect and filter.
private function Init takes nothing returns nothing
set Timer = CreateTimer()
set Rect1 = Rect(0.00,0.00,1.00,1.00)
set Tree_filt = Filter(function Check_trees)
// Map bounds
set Game_maxX = GetRectMaxX(bj_mapInitialPlayableArea) - 64.00
set Game_maxY = GetRectMaxY(bj_mapInitialPlayableArea) - 64.00
set Game_minX = GetRectMinX(bj_mapInitialPlayableArea) + 64.00
set Game_minY = GetRectMinY(bj_mapInitialPlayableArea) + 64.00
// Boolean values for destroying trees
set BA[0] = false
set BA[1] = false
// Creating unit for destroying trees
set Tree_dummy = CreateUnit(Player(PLAYER_NEUTRAL_PASSIVE),DUMMY_ID,0.00,0.00,0.00)
call SetUnitPathing(Tree_dummy,false)
call ShowUnit(Tree_dummy,false)
call UnitAddAbility(Tree_dummy,HARVEST)
call UnitAddAbility(Tree_dummy,LOCUST)
// Creating item for pathability checking
set Item = CreateItem(039;ciri039;,0.00,0.00)
call SetItemVisible(Item,false)
endfunction
endlibrary
</i></i></i></i></i>
Screenshots:
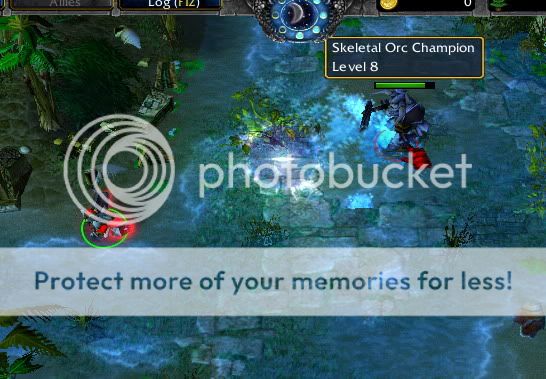
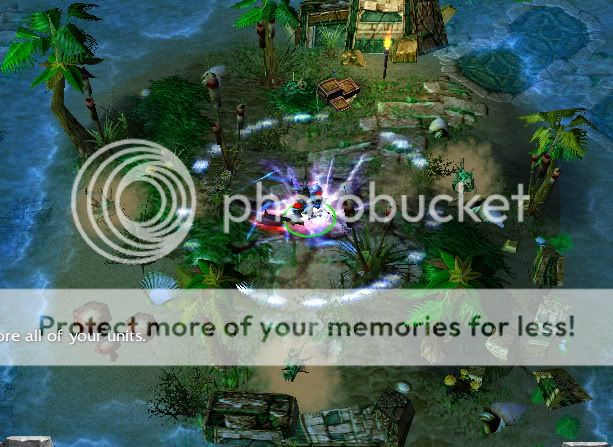
*Change Log*
- 30th Oct - Initial Release.
- 19th Nov - Version 2.00 released, few big updates to the system. Re-worked how trees are knocked down, now uses a real value instead of boolean. Read green block of writing in the code for more information. Added an option to allow the player to move the knocked back unit while it's sliding. Added an option to check terrain height changes and stop the unit accordingly. Its pretty sketchy but it gets the job done in most maps. However it has problems when there are large/steep hills in the map. Some other coding changes to increase efficiency and stuff.
- 26th Nov - Version 2.01 released. No major changes except for the addition of two simple function that were "requested" by like 1 or 2 people. So i decided to add them.
- 17th Dec - Version 2.02 released. Few major changes to how the system works, including: the parameters "Startspeed" and "Deceleration" have now been changed to "Distance" and "Duration" which i find is far easier to use. Now it is as easy as putting in a value of say 500.00 for distance and 2.00 for duration, making it last 2 seconds and knocking the unit back 500.00 distance. Also "Checkheight" (which checked for terrain height using Z locations) has now been replaced with "CheckPathing" which checks for pathable terrain using an item check. Original concept is from Vexorian, it has been slightly modified. Also added an effect for when a unit hits something with Checkpathing == true, this can also be made to not show. Unfortunately this means it is not backwards compatible with earlier versions, but this is made up for with the easier arguments needed.
- 9th April - Version 2.03 released. Few minor changes made. Standardized the coding, making it easier to read. Updated the check pathability function from Vexorian's old version to Anitarf's newer version. Few minor changes to the coding in general, changed the two bonus functions a little bit. Nothing big, but it should be a little nicer now.
- 9th April - Version 2.04 released. Another couple of minor changes. Figured out my calculation for distance was a little off, it is now fixed. An incredibly minor change to how the effects work when wanting a custom one. And finally i added a new function. Check this post for details.