quraji
zap
- Reaction score
- 144
What is VoteOwn?
Well, when I was experimenting with dialogs for another system (I never used them before...they're ugly..) I noticed an oddity. If I created a dialog and set a title, but didn't add any buttons, then showed it to myself, there was no way to close the dialog or otherwise circumvent it! It seems the only way to escape a no-button dialog is to quit the game. Naturally, I decided to abuse this and VoteOwn was born.
What does it do?
It's basically a votekick system, but instead of kicking a person, it "owns" them, by displaying to them a no-button dialog. That doesn't seem like much, but as far as I can tell the only way to remove it is to exit Warcraft III. Therefore, not only are you possibly confusing the poor noob, you're also causing him to leave the game, and actually quit Warcraft III. Ownage!
How do I use it?
Paste it into your map. Configure the variables you'd like (or don't, it's play-ready as is). That's it
Picture?
Sure:
Other stuff:
First let me say that this was to me mostly just a joke and a practice with dialogs (which are now #1 on my hatelist). So as a result, I didn't put in that extra love and care when writing this, and you'll notice it if you look at the code. But, it does work, although I'm not sure if there are any bugs yet, it's not exactly easy to test a voting system by yourself.
But, I decided to fix it up and post it, if anyone wants to use it. I might fix any minor bugs you point out, but I don't plan on updating or expanding this unless people actually take an interest in it.
A couple features of this system were disabled/removed due to a strange bug I encountered when using timers along with dialogs. For some weird reason, timers randomly stop functioning when working with dialogs. But, comment the lines dealing with dialogs and poof! the timer works again. If anyone has an explanation for this, please share it (or if I'm being stupid, point it out).
Blah, blah, blah...where's the code?
Voila:
Change it, mod it, burn it, sacrifice it to your pagan god, I don't care.
Hopefully someone will find this amusing :thup:
Disclaimer: I am not the morality police. I made the system to abuse a (possible) bug, it's up to you to use it!
(a demo map is kind of pointless for this)
Well, when I was experimenting with dialogs for another system (I never used them before...they're ugly..) I noticed an oddity. If I created a dialog and set a title, but didn't add any buttons, then showed it to myself, there was no way to close the dialog or otherwise circumvent it! It seems the only way to escape a no-button dialog is to quit the game. Naturally, I decided to abuse this and VoteOwn was born.
What does it do?
It's basically a votekick system, but instead of kicking a person, it "owns" them, by displaying to them a no-button dialog. That doesn't seem like much, but as far as I can tell the only way to remove it is to exit Warcraft III. Therefore, not only are you possibly confusing the poor noob, you're also causing him to leave the game, and actually quit Warcraft III. Ownage!
How do I use it?
Paste it into your map. Configure the variables you'd like (or don't, it's play-ready as is). That's it
Picture?
Sure:
(crappy quality due to MS Paint JPEG compression and photobucket)
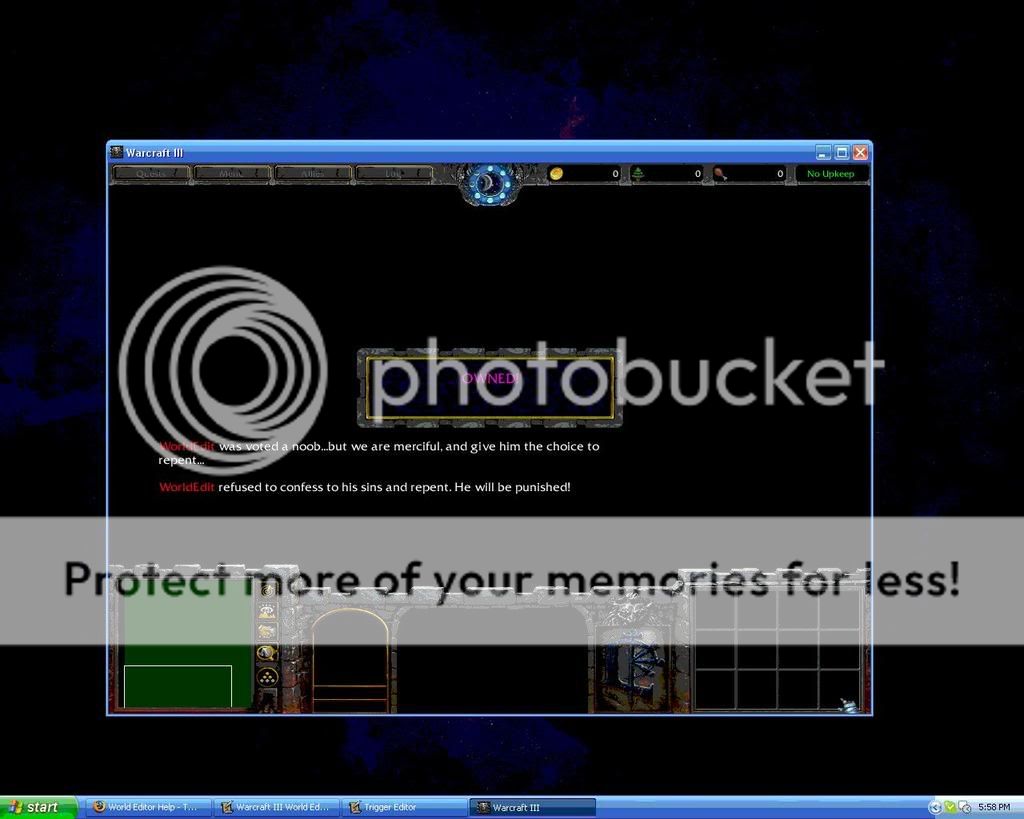
First let me say that this was to me mostly just a joke and a practice with dialogs (which are now #1 on my hatelist). So as a result, I didn't put in that extra love and care when writing this, and you'll notice it if you look at the code. But, it does work, although I'm not sure if there are any bugs yet, it's not exactly easy to test a voting system by yourself.
But, I decided to fix it up and post it, if anyone wants to use it. I might fix any minor bugs you point out, but I don't plan on updating or expanding this unless people actually take an interest in it.
A couple features of this system were disabled/removed due to a strange bug I encountered when using timers along with dialogs. For some weird reason, timers randomly stop functioning when working with dialogs. But, comment the lines dealing with dialogs and poof! the timer works again. If anyone has an explanation for this, please share it (or if I'm being stupid, point it out).
Blah, blah, blah...where's the code?
Voila:
JASS:
library VoteOwn initializer Init
// by quraji, Master Ownerer
// Configurables
globals
private constant string VoteOwn_Command = "-vo"
private constant real Vote_Threshold = .66 // percent of people that need to vote for owning the noob, to own the noob
private constant boolean Repent_Allow = true // allow the noob to repent (give him a second chance, next time only half the threshold number of votes are required)
private constant real Vote_Time = 10. // the time to vote (vote auto-ends once time runs out)
private constant real Repentance_Time = 1. // the time the player has to repent (defaults to No if the player doesn't respond in this time)
private constant real NoVote_Time = 10. // the time after a vote session that no votes may be issued.
public boolean VoteOwn_Allow = true // the public global you may set as you wish, false means no voting allowed
endglobals
// Message Constants
globals
private constant string VoteOwn_NotAllowed = "VoteOwning is not allowed at this time." // message to display if VoteOwning is turned off and someone tries to VoteOwn
// OWNED!
private constant string Owned_Colour = "|cffff00e0" // a nice pretty pink to put the noob in his place
private constant string Owned_Message = Owned_Colour + "OWNED!" + "|r" // message to impart upon the noob
// OWNED!
private constant string ToNoob_Safe = "" // if the player is not voted to get owned, display this message to them
private constant string ToNoob_Unsafe = "" // if the player is voted to get owned, display this to them
private constant string ToPlayers_VoteFail = "" // message to show players if the vote fails
private constant string ToPlayers_VoteSuccess = "" // message to show players if vote is a success
private constant string ToPlayers_RepentStart = "" // message to show players if the player is given option to repent
private constant string ToPlayers_RepentYes = "" // message to show players if the player repents
private constant string ToPlayers_RepentNo = "" // message to show if player does not repent
private constant string Title_Colour = "|cffc8b9b6" // easy on the eyes
private constant string VoteStart_Title = Title_Colour + "Who039;s the noob?" + "|r" // I don't think the colour will carry but return just to be safe
private constant string VoteStart_NoVote = "|cffffffffNevermind|r"
private constant string Vote_Title = "" // auto-assigns a title if this is "", recommended to leave it
private constant string Vote_Yes = "|cffffffffYay|r"
private constant string Vote_No = "|cffffffffNay|r"
private constant string Vote_NoVote = "|cffbAbAbANo Vote|r"
private constant string Repent_Title = Title_Colour + "You have been found guilty |nof Noobdom! Will you repent?" // remember to use a linebreak (|n) if your message is too long or the text
// will spill over the sides of the dialog box, making it even uglier than it is
private constant string Repent_Yes = "|cffffffffI repent!|r"
private constant string Repent_No = "|cffbAbAbANever!|r"
endglobals
// Dialogs
globals
private dialog Punisher = DialogCreate()
private dialog Dialog_VoteStart = DialogCreate()
private button array VoteStart_Buttons [13]
private dialog Dialog_Vote = DialogCreate()
private button array Vote_Buttons [3]
private dialog Dialog_Repent = DialogCreate()
private button array Repent_Buttons [2]
endglobals
// Other Stuff
globals
private string array PlayerColours [12]
private trigger VoteStart_Trig = CreateTrigger()
private trigger Vote_Trig = CreateTrigger()
private trigger Repent_Trig = CreateTrigger()
private player CurrentNoob
private player CurrentOwnerer
private boolean array HasRepented [12]
private integer CurrentVotes = 0 // number of votes collected
private integer VotesFor = 0 // number of votes for owning the noob
private timer Vote_Timer = CreateTimer()
private boolean AllowVoting = true
private boolean VoteInProgress = false
endglobals
private function PunishNoob takes nothing returns nothing
call DialogSetMessage(Punisher, Owned_Message)
call DialogDisplay(CurrentNoob, Punisher, true)
endfunction
private function AllowVoting_Flip takes nothing returns nothing
set AllowVoting = not AllowVoting
endfunction
private function Repent_Response takes nothing returns nothing
local button b = GetClickedButton()
local string s
local string t = PlayerColours[GetPlayerId(CurrentNoob)] + GetPlayerName(CurrentNoob) + "|r"
if b == Repent_Buttons[0] then
set s = ToPlayers_RepentYes
// ToPlayers_RepentYes isn't set, auto-assign
if s == "" then
set s = t + " has repented, and forsaken the path of noobdom. Punishment will come swiftly if he falls astray once again."
endif
// remember that the player has repented
set HasRepented[GetPlayerId(CurrentNoob)] = true
else
set s = ToPlayers_RepentNo
// ToPlayers_RepentNo isn't set, auto-assign
if s == "" then
set s = t + " refused to confess to his sins and repent. He will be punished!"
endif
call PunishNoob()
endif
set b = null
call TimerStart(Vote_Timer, NoVote_Time, false, function AllowVoting_Flip)
call DisplayTimedTextToPlayer(GetLocalPlayer(), 0., 0., 10., s)
endfunction
private function Vote_End takes nothing returns nothing
local string s = " "
local string t
local string c = PlayerColours[GetPlayerId(CurrentNoob)]
call PauseTimer(Vote_Timer)
// remove the dialogs, in case someone didn't vote
call DialogDisplay(GetLocalPlayer(), Dialog_Vote, false)
// if not enough people voted yes (remember to add the starting voter's vote)
if ((VotesFor+1) / (CurrentVotes+1) < Vote_Threshold) or (HasRepented[GetPlayerId(CurrentNoob)] and ((VotesFor+1)*2) / (CurrentVotes + 1) < Vote_Threshold) then
set s = ToNoob_Safe
// ToNoob_Safe isn't set, auto-assign
if s == "" then
set s = c + "You|r are safe...for now."
endif
set t = ToPlayers_VoteFail
// ToPlayersFail isn't set, auto-assign
if t == "" then
set t = "Voting has concluded, and the result is rather boring. " + c + GetPlayerName(CurrentNoob) + "|r was not voted a noob, lucky him."
endif
call TimerStart(Vote_Timer, NoVote_Time, false, function AllowVoting_Flip)
else
if Repent_Allow and HasRepented[GetPlayerId(CurrentNoob)] == false then
set t = ToPlayers_RepentStart
// ToPlayers_RepentStart isn't set, auto-assign
if t == "" then
set t = c + GetPlayerName(CurrentNoob) + "|r was voted a noob...but we are merciful, and give him the choice to repent..."
endif
call DialogSetMessage(Dialog_Repent, Repent_Title)
call DialogDisplay(CurrentNoob, Dialog_Repent, true)
else
set s = ToNoob_Unsafe
// ToNoob_Unsafe isn't set, auto-assign
if s == "" then
set s = c + "You|r are the weakest link, goodbye!"
endif
set t = ToPlayers_VoteSuccess
// ToPlayers_Success isn't set, auto-assign
if t == "" then
set t = "The people have spoken. " + c + GetPlayerName(CurrentNoob) + "|r is a noob, and will be punished!"
endif
call PunishNoob()
call TimerStart(Vote_Timer, NoVote_Time, false, function AllowVoting_Flip)
endif
endif
set VoteInProgress = false
call DisplayTimedTextToPlayer(GetLocalPlayer(), 0., 0., 10., t)
call DisplayTimedTextToPlayer(CurrentNoob, 0., 0., 8., s)
endfunction
private function Vote_Response takes nothing returns nothing
local button b = GetClickedButton()
local integer i = 0
local integer j = 0
// player clicked NoVote, exit
if b == Vote_Buttons[2] then
return
// player clicked Yes, increment number of votes for owning
elseif b == Vote_Buttons[0] then
set VotesFor = VotesFor + 1
endif
set b = null
// increment number of votes
set CurrentVotes = CurrentVotes + 1
// count active players
loop
exitwhen i == 12
if GetPlayerSlotState(Player(i)) == PLAYER_SLOT_STATE_PLAYING then
set j = j + 1
endif
set i = i + 1
endloop
//if all players (minus the starter and noob) have voted, end the vote
if CurrentVotes == j then//-2 then
call Vote_End()
endif
endfunction
private function VoteStart_Response takes nothing returns nothing
local button b = GetClickedButton()
local integer i = 0
local integer j = 0
local string s
local player p = GetLocalPlayer()
// player clicked the NoVote button, so exit
if b == VoteStart_Buttons[13] then
set AllowVoting = true
return
// player is trying to be a smartass and vote for himself
elseif false then //b == VoteStart_Buttons[GetPlayerId(CurrentOwnerer)] then
call DisplayTimedTextToPlayer(CurrentOwnerer, 0., 0., 6., "Don039;t be a smartass.")
set AllowVoting = true
return
endif
set VoteInProgress = true
set CurrentVotes = 0
set VotesFor = 0
loop
exitwhen i == 12
if b == VoteStart_Buttons<i> then
set CurrentNoob = Player(i)
set j = i
endif
set i = i + 1
endloop
set b = null
set s = Vote_Title
// Vote_Title isn't set, auto-assign
if s == "" then
set s = Title_Colour + "Is|r " + PlayerColours[j] + GetPlayerName(CurrentNoob) + "|r" + Title_Colour + "|n a noob?|r"
endif
call DialogSetMessage(Dialog_Vote, s)
//only display the voting dialog to people who aren't the noob/ownerer
//if p != CurrentNoob and p != CurrentOwnerer then
call DialogDisplay(p, Dialog_Vote, true)
//endif
set p = null
// add a timer to automatically end the vote, in case players are sleeping
call TimerStart(Vote_Timer, Vote_Time, false, function Vote_End)
endfunction
private function PlayerEnteredCommand takes nothing returns nothing
local string s
if VoteOwn_Allow == true and AllowVoting == true then
set CurrentOwnerer = GetTriggerPlayer()
set AllowVoting = false
call DialogSetMessage(Dialog_VoteStart, VoteStart_Title) // set dialog title here because it doesn't like being set at map init
call DialogDisplay(CurrentOwnerer, Dialog_VoteStart, true)
elseif VoteInProgress then
call DisplayTextToPlayer(GetTriggerPlayer(), 0., 0., "A vote is already in progress!")
elseif AllowVoting == false then
set s = I2S(R2I(TimerGetRemaining(Vote_Timer)))
call DisplayTextToPlayer(GetTriggerPlayer(), 0., 0., "VoteOwning is disabled temporarily, please wait " + s + " seconds, and try again.")
else
call DisplayTextToPlayer(GetTriggerPlayer(), 0., 0., VoteOwn_NotAllowed)
endif
endfunction
private function Init takes nothing returns nothing
local trigger t = CreateTrigger()
local integer i = 0
local integer j = 0
local string s
loop
exitwhen i == 12
call TriggerRegisterPlayerChatEvent(t, Player(i), VoteOwn_Command, true)
set i = i + 1
endloop
call TriggerAddAction(t, function PlayerEnteredCommand)
set t = null
// PlayerColours setup
set PlayerColours[0] = "|cffff0000" // red
set PlayerColours[1] = "|cff0000ff" // blue
set PlayerColours[2] = "|cff00ffff" // cyan
set PlayerColours[3] = "|cff551a8b" // purple
set PlayerColours[4] = "|cffffff00" // yellow
set PlayerColours[5] = "|cffffa500" // orange
set PlayerColours[6] = "|cff00ff00" // green
set PlayerColours[7] = "|cffff00ff" // magenta
set PlayerColours[8] = "|cffbebebe" // grey (anyone who says "It's spelled gray!" is getting slapped, they're both acceptable)
set PlayerColours[9] = "|cff00bfff" // light blue
set PlayerColours[10] = "|cff006400" // dark green
set PlayerColours[11] = "|cff8b2323" // brown
// end PlayerColours setup
loop
exitwhen j == 12
if GetPlayerSlotState(Player(j)) == PLAYER_SLOT_STATE_PLAYING then
set s = PlayerColours[j] + GetPlayerName(Player(j)) + "|r"
set VoteStart_Buttons[j] = DialogAddButton(Dialog_VoteStart, s, 0)
endif
set j = j + 1
endloop
set VoteStart_Buttons[13] = DialogAddButton(Dialog_VoteStart, VoteStart_NoVote, 0)
// Dialog_Vote buttons
set Vote_Buttons[0] = DialogAddButton(Dialog_Vote, Vote_Yes, 0)
set Vote_Buttons[1] = DialogAddButton(Dialog_Vote, Vote_No, 0)
set Vote_Buttons[2] = DialogAddButton(Dialog_Vote, Vote_NoVote, 0)
// Dialog_Repent buttons
set Repent_Buttons[0] = DialogAddButton(Dialog_Repent, Repent_Yes, 0)
set Repent_Buttons[1] = DialogAddButton(Dialog_Repent, Repent_No, 0)
// VoteStart_Trig Setup
call TriggerRegisterDialogEvent(VoteStart_Trig, Dialog_VoteStart)
call TriggerAddAction(VoteStart_Trig, function VoteStart_Response)
// Vote_Trig Setup
call TriggerRegisterDialogEvent(Vote_Trig, Dialog_Vote)
call TriggerAddAction(Vote_Trig, function Vote_Response)
// Repent_Trig Setup
call TriggerRegisterDialogEvent(Repent_Trig, Dialog_Repent)
call TriggerAddAction(Repent_Trig, function Repent_Response)
endfunction
endlibrary</i>
Change it, mod it, burn it, sacrifice it to your pagan god, I don't care.
Hopefully someone will find this amusing :thup:
Disclaimer: I am not the morality police. I made the system to abuse a (possible) bug, it's up to you to use it!
(a demo map is kind of pointless for this)