I
IKilledKEnny
Guest
This is my newly made JASS spell, "Burning Steps". Took me about 20 minutes to make it, but I still thinks it's decent. 
What does it do:
Once the spell have been casted it will last for 5 / 10 / 15 seconds. Druing that time
The hero's speed will increase by 20% / 30% / 35%, also after the hero there will be a
trail of flames left, each flame created will cost 0.5 / 1 / 1.5 mana for the caster.
When an enemy unit will step into the flames, it will be dealt damage of 15 / 20 / 30 per
second. Once created flames will be removed only after 4 / 5 / 6 seconds.
The Trigger:
This is the READ ME:
Enjoy, and please comment.
What does it do:
Once the spell have been casted it will last for 5 / 10 / 15 seconds. Druing that time
The hero's speed will increase by 20% / 30% / 35%, also after the hero there will be a
trail of flames left, each flame created will cost 0.5 / 1 / 1.5 mana for the caster.
When an enemy unit will step into the flames, it will be dealt damage of 15 / 20 / 30 per
second. Once created flames will be removed only after 4 / 5 / 6 seconds.
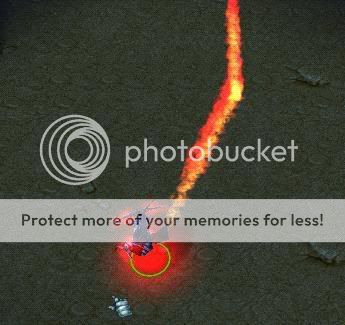
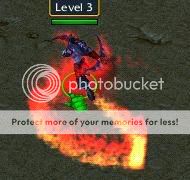
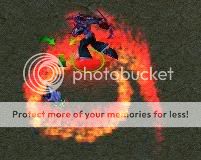
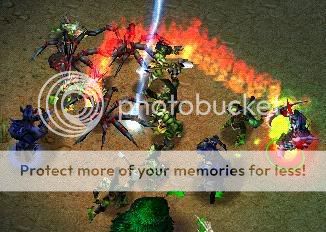
The Trigger:
JASS:
constant function BurningStepsId takes nothing returns integer
// This function will return the base Id of the burning steps spell.
return 039;A000039;
// You need to edit 'A000' to burning step's Id in your map.
endfunction
constant function BurningStepsImmolationId takes nothing returns integer
// This function will return the base Id of the burning steps immolation spell.
return 039;A001039;
// You need to edit 'A001' to burning steps immolation's Id in your map.
endfunction
constant function BurningStepsDummyId takes nothing returns integer
// This function will return the base Id of the dummy.
return 039;h000039;
// You need to edit 'h000' to dummy's Id in your map.
endfunction
function BurningStepsDummyTime takes integer abilitylevel returns real
// This function will return the life time of the flames
return I2R(abilitylevel * 3 + 1)
// At the moment it returns 4 / 5 / 6.
endfunction
constant function BurningStepsRedo takes unit caster returns real
// This function will return the time which will take the timer to run the new function
return (20/GetUnitMoveSpeed(caster))
// I suggest making this equal to immolation AoE divided by move speed although it's not a have to.
endfunction
function BurningStepsWaitTime takes integer abilitylevel returns real
// This function will return the dauration of the spell
return I2R(abilitylevel*5)
// At the moment it returns 5 / 10 / 15.
endfunction
constant function BurningStepsNewMana takes real mana, integer abilitylevel returns real
// This function will return the mana cost per flamce
return mana-abilitylevel*0.5
// At the moment it's current mana - .5 / 1 / 1.5
endfunction
function BurningStepsCon takes nothing returns boolean
// This function will return true if the spell that was caster is burning steps.
return GetSpellAbilityId()==BurningStepsId()
// If this returns flase the action triggers will not run.
endfunction
function BurningSteps takes nothing returns nothing
// This function will create the flames at the hero's feet
local timer caller=GetExpiredTimer() // The timer which triggered the function
local unit caster=GetHandleUnit(caller,"caster") // We get the caster through it's link with the timer
local real casterX=GetUnitX(caster) // We get the unit's X. X + Y = location
local real casterY=GetUnitY(caster) // We get the unit's Y. X + Y = location
local player owner=GetOwningPlayer(caster) // We get the owner of the caster
local unit FlameDummy // This will serve as the flame when the time comes
local integer AbilityLevel=GetUnitAbilityLevel(caster,BurningStepsId()) // This gets the level of burning steps for the caster
local real mana=GetUnitState(caster, UNIT_STATE_MANA) // This get's the caster's current mana
local real NewMana=BurningStepsNewMana(mana,AbilityLevel) // This get's it's new mana
local real counter=GetHandleReal(caller,"counter") // We get counter through it's link with the timer
local real adder=GetHandleReal(caller,"adder") // We get adder through it's link with the timer
local real cap=GetHandleReal(caller,"cap") // We get cap through it's link with the timer
set counter=counter+adder // We set the new value of counter
if counter >=cap then // We check if it is equal to cap or bigger
call PauseTimer(caller) // We are pausing the timer so we could destroy safely.
call FlushHandleLocals(caller) // We destroy the linkage between caster and timer
call DestroyTimer(caller) // We destroy timer
// Leaks removal (we null stuff)
set FlameDummy=null
set owner=null
set caller=null
set caster=null
endif
if NewMana > 0 then // We check if the caster's new mana is more then 0 if it is then (else we do nothing):
set FlameDummy=CreateUnit(owner,BurningStepsDummyId(),casterX,casterY,0) // This creates a flame for owner in the caster's location.
call SetUnitState(caster,UNIT_STATE_MANA, NewMana) // This set's the caster's mana to NewMana
call UnitAddAbility(FlameDummy,BurningStepsImmolationId()) // We add immolation to the flame
call SetUnitAbilityLevel(FlameDummy,BurningStepsImmolationId(),AbilityLevel) // We set immolation's level to the burning step's level for the caster.
call UnitApplyTimedLife(FlameDummy,039;BTLF039;,BurningStepsDummyTime(AbilityLevel)) // We add timed life to the flame so it will not live forever.
endif
call SetHandleReal(caller,"counter",counter)
// Leaks removal, nulling stuff
set FlameDummy=null
set owner=null
set caller=null
set caster=null
endfunction
function BurningStepsMain takes nothing returns nothing
// This the main function that will be triggered when the caster cast's burning steps
local timer caller=CreateTimer() // We create a timer
local unit caster=GetTriggerUnit() // We get triggering unit
local real redo=BurningStepsRedo(caster) // We get the time between each function call of function Burning Steps.
local integer AbilityLevel=GetUnitAbilityLevel(caster,BurningStepsId()) // We get the level of burning steps for the caster
local real cap=BurningStepsWaitTime(AbilityLevel) // We set the spell's dauration
local real adder=redo // This will be used for stoping the spell at the right time. We will increase counter by the adder (which is redo).
local real counter=0 // We will use counter to count number of seconds the spell is running and if it ran too much, then we will stop it.
call SetHandleHandle(caller,"caster",caster) // We create linkage between caster and timer
call SetHandleReal(caller,"counter",counter) // We create linkage between counter and timer
call SetHandleReal(caller,"adder",adder) // We create linkage between adder and timer
call SetHandleReal(caller,"cap",cap) // We create linkage between cap and timer
call TimerStart(caller,redo,true,function BurningSteps) // We start timer that will run every "redo" time and will call BurningSteps function.
set caster=null
endfunction
//===========================================================================
function InitTrig_BurningSteps takes nothing returns nothing
// This function will create, and then set the trigger.
set gg_trg_BurningSteps=CreateTrigger()
call TriggerRegisterAnyUnitEventBJ(gg_trg_BurningSteps,EVENT_PLAYER_UNIT_SPELL_EFFECT)
call TriggerAddCondition(gg_trg_BurningSteps,Condition(function BurningStepsCon))
call TriggerAddAction(gg_trg_BurningSteps,function BurningStepsMain)
endfunction
This is the READ ME:
Code:
Spell made by ~IKilledKenny~
================================================================================================
>> Please follow these rules:
> You may import this spell freely into your map, with no credits needed, so don't worry
about that. However, when importing it, make sure you follow the importing instructions
or problems may occur becuase of this spell.
> While you may use this spell freely in your maps, please don't state in another websites
that this was made by you. It's called stealing, and usually it is not moral to do so.
================================================================================================
>> Description:
> Once the spell have been casted it will last for 5 / 10 / 15 seconds. Druing that time
The hero's speed will increase by 20% / 30% / 35%, also after the hero there will be a
trail of flames left, each flame created will cost 0.5 / 1 / 1.5 mana for the caster.
When an enemy unit will step into the flames, it will be dealt damage of 15 / 20 / 30 per
second. Once created flames will be removed only after 4 / 5 / 6 seconds.
================================================================================================
>> The Trigger:
> The trigger is made of many functions. There will be 7 functions that are used for
editing values of the spell (flame's life time, spell Id and so on), 5 of them are
constant. Those 7 functions will be used by the last 4 which will use them in order
to define actions, conditions and events.
> If you are having a hard time reading the trigger for any reason, read the detailed
comments around it which say what each line does. Comments are written after "//".
> Note: I'm using KaTTaNa's handle system, but only part of the functions, not all. The
vesion I'm using of the Handle System is located in the header of the map.
================================================================================================
>>> The map:
>> In the map there will be certain helpful features which will aid you in testing the
spell and having fun with it. The features will be triggered by typing in a certain
command, here is the least of all features that they can be found in the map and how
they will be triggered:
> "level": Will increase your hero's level by one.
> "creeps": This will create few creeps that will serve as training puppets.
> "revive: If your hero died, typing revive will revive him.
> "heal": Will get you mana and health values to the maximum.
>> There is no need to copy those triggers, they are there just to help you while testing
the spell in this template. The map triggers differently from the spell are made in GUI
and they leak as well.
================================================================================================
>>> The spells, buffes and units.
>> Spells
> Burning Steps is based on berserk. Burning Steps can be found in the object editor
under Spells > Custom Abilities > Human > Hero.
> Immolation (Burning Steps) is based on Permanent Immolation and can be found in the
the object editor under Spells > Custom Abilities > Human > Units.
>> Buffes
> Burning steps can be found in the object editor > Buffs > Custom buffs > Human
> Units.
> Burning steps (immolation) can be found in the object editor > Buffs > Custom buffs
> Special > Units
>> Units
> The demon (the tester) can be found under units > Custom units > Humans > Melee
>Heros.
> The dummy can be found under units > Custom units > Humans > Melee > Units.
================================================================================================
>> Importing Instructions
> Firstly, go on and import both buffs, their location can be found above. You can copy
buffs my clicking ctrl + c on them and then going into your buffs manager in your map and
pressing ctrl + v.
> After you have done that, copy both spells, their location can be found above. You can
copy spells my clicking ctrl + c on them and then going into your spellss manager in your
map and pressing ctrl + v.
> Now you need to copy the dummy unit, you may also copy the demon if you want, but you don't
have to, their location can be found above. You can copy units by clicking ctrl + c on
them and then going into your units manager in your map and pressing ctrl + v.
> After you copied everything from the object editor into your map go to the map header
(where it says Burning Steps.w3x) and copy everything in there into your map header. Make
sure you copy everything there.
> After all those steps have been done of create new trigger named "BurningSteps" (case
sensitive) and copy every bit of the tirgger to it.
> Lastly go to the object editor and click on ctrl + d, you could see the raw data of
everything. Using this information edit the dummy's, spell's and immolation's Ids in the
trigger so it won't refer to other handles by mistake.
================================================================================================
>> Last words
> If you have any suggestion, questions or bugs found you can contact me via private
massage in thehelper.net webiste, nickname IKilledKEnny. If you wish you can also send
E-mail to [email protected].
> Enjoy!
Your favoriate murderer,
IKilledKenny
Enjoy, and please comment.